Overview
JxMusic is for those who prefer to use a desktop app for listening to music. More importantly, JxMusic is optimized for those who prefer to work with a Command Line Interface (CLI) while still having the benefits of a Graphical User Interface (GUI).
Summary of contributions
-
Major enhancement: Storage component of Jxmusic
-
What it does: Enable the app to save the Library’s Playlist information into JSON file, read from library to initialize library class, and scan all valid mp3 files in the /library folder.
-
Justification: It is important in this application because it allows the user to have their playlists information stored. Furthermore, it loads the library folder’s mp3 files and display them on the track panel.
-
Highlights: This enhancement takes the edge cases into considerations when the library folder is empty or does not exist, it can extract a default library from resource folder of the application.
-
-
Other Contributions:
-
Minor enhancements: Added Track List Command and Track Search Command, which allow users to navigate the all the tracks available in the library folder, and search tracks using keywords.
-
Contributions to project management:
-
Evidence of helping others:
-
Spotted bugs and edge cases on the track and playlist search command.
-
Found bugs on the Group T12-4’s software. #153
-
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Listing all tracks : track list
Shows a list of all tracks in the library.
Format: track list
Stepping a track : step
[coming in v2.0]
Steps forward by default 10 seconds or specified time in seconds.
Format: step [s/SECONDS]
Examples:
-
play t/Some Song
step
The track moves forward to 10 seconds later of the track and keeps playing. -
play t/Some Song
step s/100
Stepping forward 100 seconds. -
play t/Some Song
stop s/-100
Using negative value forSECONDS
will apply the same behaviour asstepback
. The track steps backward 100 seconds.
Stepping back : stepback
[coming in v2.0]
Steps back by default 10 seconds or specified time in seconds.
Format: stepback [s/SECONDS]
Examples:
-
play t/Some Song
stepback
The track moves backward 10 seconds earlier of the track and keeps playing. If the track has just played for less than 10 seconds, the track replays. -
play t/Some Song
stepback s/100
Stepping back 100 seconds. -
play t/Some Song
stepback s/-100
Using negative value forSECONDS
will apply the same behaviour asstep
. The track steps forward 100 seconds.
Replay a track : replay
[coming in v2.0]
Replays a track before the track ends to start from the beginning.
Format: replay
Examples:
-
play t/Some Song
seek t/100
replay
The track plays from the beginning.
Skipping to the next track : next
[coming in v2.0]
Plays the next track.
Format: next
Examples:
-
play p/Favourites
next
If the last track is being played, the playlist ends and stops. -
play t/Some Song
repeat track
next
“Some Song” will replay. -
play p/Favourites
repeat playlist
next
Go to the next track. Sincerepeat playlist
is on, if it is the last track of the playlist playing, the first track of the playlist will play. -
play p/Favourites
shuffle
repeat playlist
next
Go to the next track. Sinceshuffle
andrepeat playlist
are on, if it is the last track playing, the next shuffled repeat will play.
Skipping to the previous track : prev
[coming in v2.0]
Plays the previous track.
Format: prev
Examples:
-
play p/Favourites
prev
Plays the previous track. If the current track is first track in playlist, replays the same track since there is no previous track. -
play t/Some Song
repeat track
prev
“Some Song” will replay. -
play p/Favourites
repeat playlist
prev
Go to the previous track. Sincerepeat playlist
is on, if it is the first track of the playlist playing, the last track of the playlist will play. -
play p/Favourites
shuffle
repeat playlist
prev
Go to the previous track. Sinceshuffle
andrepeat playlist
are on, if it is the first track playing and there was no previous track, the same track repeats.
Repeatedly playing a track : repeat track
[coming in v2.0]
Switches the repeat mode to repeatedly play a single track. The command works even without any track playing. Upon running this command, any subsequent track will be played on repeat.
Format: repeat track
Examples:
-
play p/Favourites
repeat playlist
repeat track
Switches to repeat track mode. The currently playing track will be on repeat. -
repeat track
play t/Some Song
“Some Song” will play on repeat. -
repeat track
play p/Favourites
The first track of the “Favourites” playlist will play on repeat.
Repeatedly playing a playlist : repeat playlist
[coming in v2.0]
Switches the repeat mode to repeatedly play a single playlist. The command works even without any playlist playing. Upon running this command, any subsequent playlist will be played on repeat.
Format: repeat playlist
Examples:
-
play p/Favourites
repeat track
repeat playlist
Switches to repeat playlist mode. “Favourites” will play on repeat. -
repeat playlist
play p/Favourites
“Favourites” will play on repeat. -
repeat playlist
play t/Some Song
“Some Song” will play on repeat.
Turning off repeat mode : repeat off
[coming in v2.0]
Turns off the repeat mode
Format: repeat off
Examples:
-
play p/Favourites
repeat playlist
repeat off
Switches off repeating mode.
Shuffling a playlist : shuffle
[coming in v2.0]
Switches on shuffle mode to play tracks in random order.
Format: shuffle
Examples:
-
shuffle
play p/Favourites
“Favourites” will play in random order. -
play p/Favourites
repeat playlist
shuffle
Subsequent tracks will be played in random order. When all the tracks in the playlist has been played, the next repeat will be shuffled. -
play p/Favourites
repeat track
shuffle
The track being repeated will continue to play untilrepeat off
only then subsequent tracks will play in random order from the remaining unplayed tracks in the playlist.
Turning off shuffle mode : shuffle off
[coming in v2.0]
Turns off the shuffle mode
Format: shuffle off
Examples:
-
play p/Favourites
shuffle
shuffle off
Switches off shuffling mode.
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
ReadLibrary
Current Implementation
The readLibrary
is a part of JsonLibraryStorage
. It reads the Json file as well as the library folder when initialising the library
class. It calls the following operations:
-
JsonFileStorage.loadDataFromFile(filepath)
- deserialize the Json file and createlibrary
class. -
Trackscanner.scan(libraryDir)
- scan through the library folder and fill all the valid mp3 files into a set of tracks.
No matter whether the Json file exists, trackscanner
will run first to make sure the library
class contains the tracks set. Then if the file path exists, it will deserialize the json file to initialize library’s playlist information. The deserializer and serializer are programmed with Gson
library.
The trackscanner
navigates to the library folder and extracts the mp3 files and add them to the set of tracks. However there are cases that the user does not have a library folder in the same directory as the app. To ensure the tracks contained inside the playlist to be valid and navigable, we put a library folder containing default tracks inside the resources folder.
Hence, the program first extracts the library folder from the jar file and copy it to the directory. There are two methods that the app copies library to the directory:
-
Case 1: If the MainApp is run using jar file,
trackscanner
will internally run the unzip command to unzip the jar file. (Warning: This only works if the jar file is opened using Terminal); -
Case 2: If the MainApp is run using IDE,
trackscanner
will copy the library folder from resources to the directory;
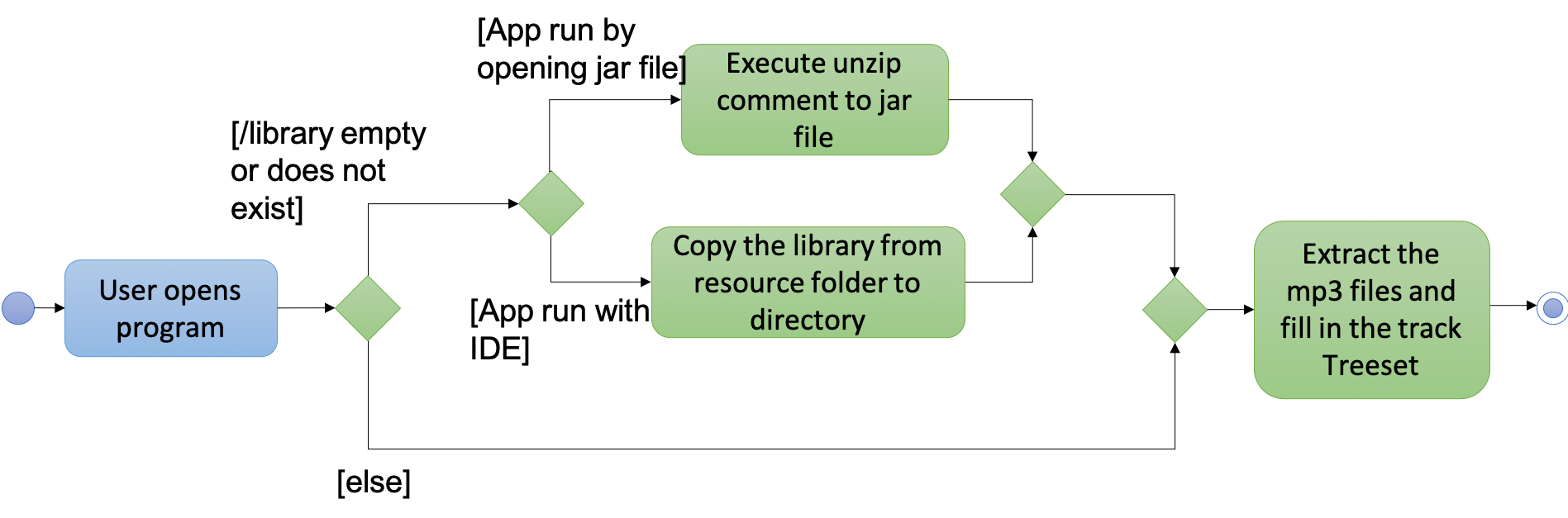
Design Considerations
Aspect: Choice of media player library
-
Alternative 1 (current choice): Read the Json file from data folder and scan the tracks from library folder at the same time.
-
Pros:
-
Enables the library to initialise with all the available tracks in library folder
-
-
Cons:
-
The track treeset in the library class will not update again after initialisation.
-
-
-
Alternative 2: Do not scan the tracks in
readLibrary
, scan the library each time the user search for tracks in the library folder.-
Pros:
-
Lead to the files’ latest status inside the library folder
-
-
Future Enhancements
When the user double clicks the jar file it cannot run because the unzipping of jar file is done using process.exec
, which requires the user to run the jar file using terminal. This needs to be addressed in future development.
Track search and Track list
Current Implementation
The tracklist
and tracksearch
commands are implemented to view and filter tracks in the library.
-
Command.TrackListCommand
- list all the tracks inside the library -
Command.TrackSearchCommand
- search the desired tracks from library whose name contains any of the argument keywords.
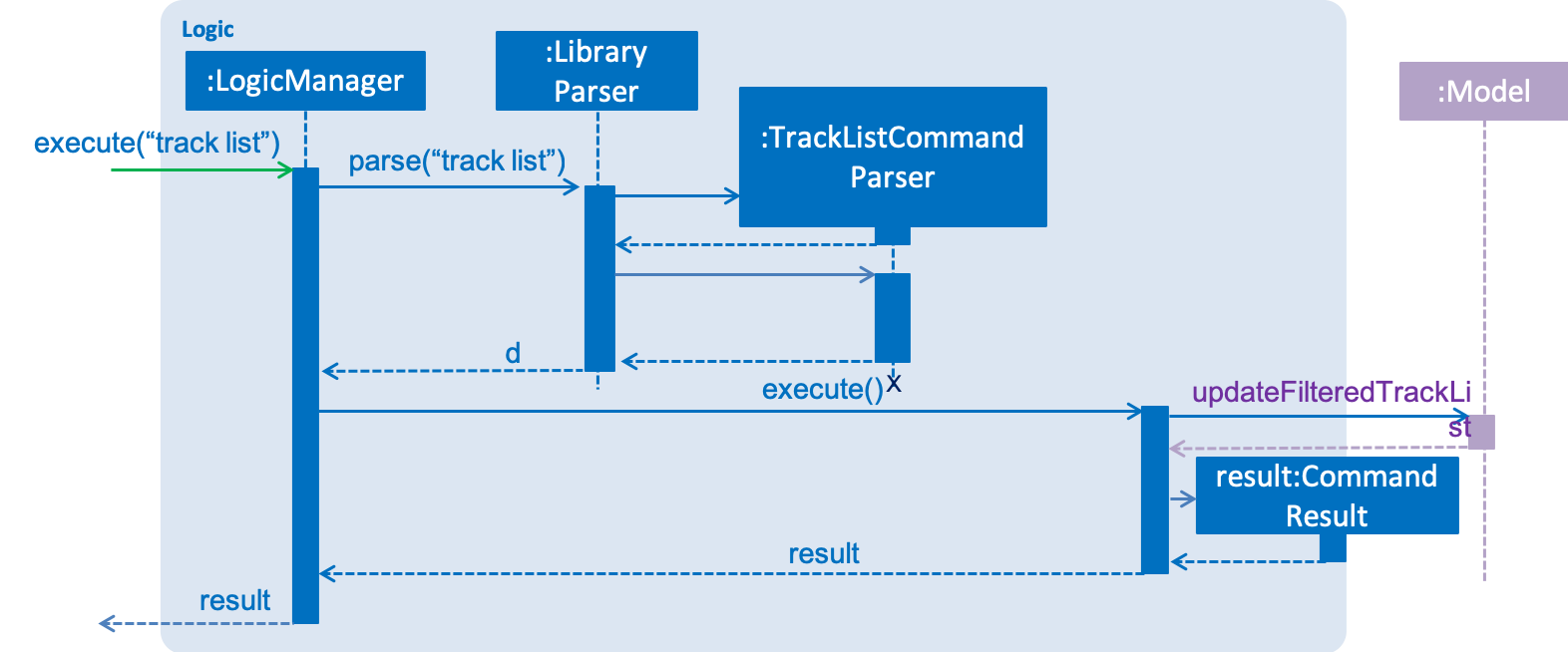
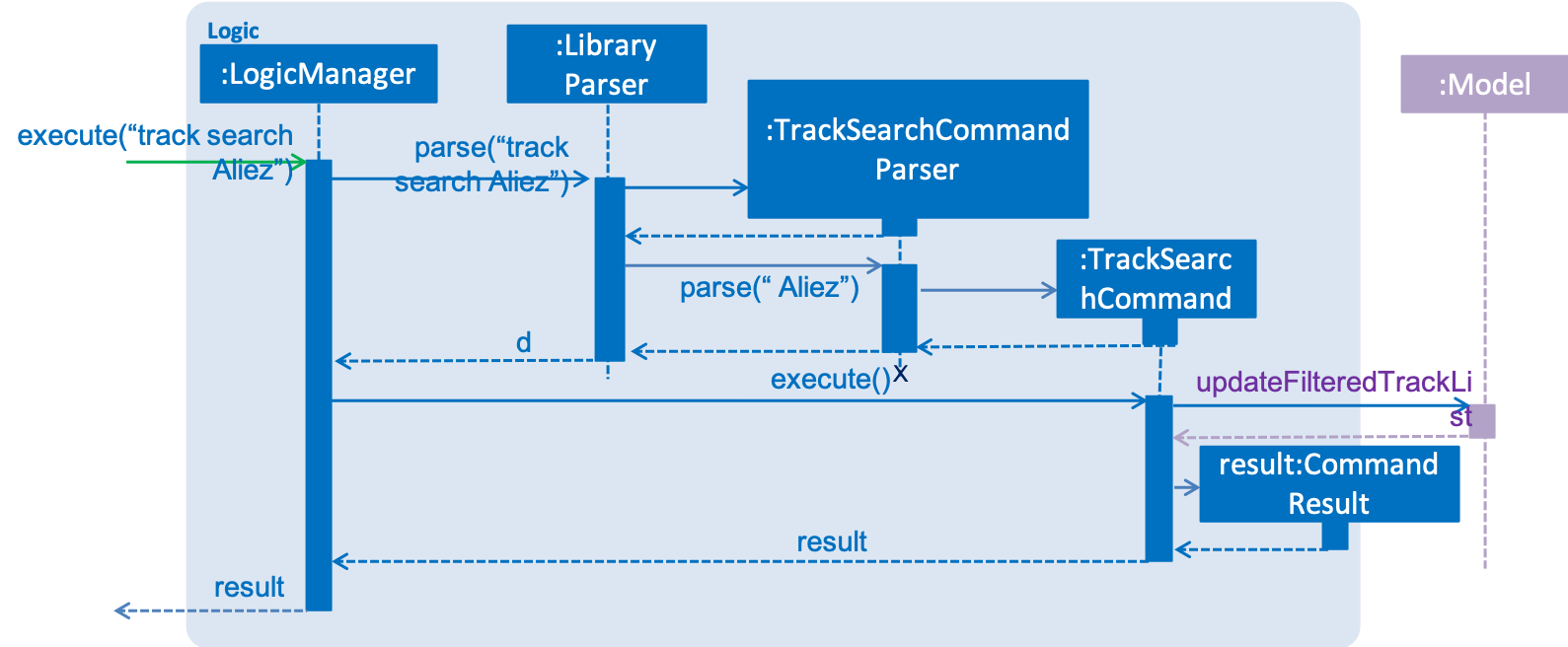
Design Considerations
Aspect: Searching multiple keywords.
-
Alternative 1 (current choice): Combines each search results by each keywords separated by space.
-
Pros:
-
Find tracks that match with any of the keywords
-
-
Cons:
-
Spaces are not included in the keywords.
-
-
-
Alternative 2: consider words as one part of the desired track and show results of the search.
-
Pros:
-
Show more precise results
-
-
Aspect: Tracks listed display
-
Alternative 1 (current choice): List the tracks in a new panel separate from playlist panel.
-
Pros:
-
Tracks are more clearly shown.
-
-
-
Alternative 2: Show the tracks in the message box
-
Pros:
-
Easier to implement.
-
-
Cons:
-
Takes up the message box and hence the success message or failing message cannot be seen.
-
-
Future Enhancements
Future development can combine the two alternatives so that the user can search with keywords including spaces when they intend to.