By: CS2103-AY1819S1-T13-3
Since: Aug 2018
Licence: MIT
- 1. Setting up
- 2. Design
- 3. Implementation
- 4. Documentation
- 5. Testing
- 6. Dev Ops
- Appendix A: Product Scope
- Appendix B: User Stories
- Appendix C: Use Cases
- Appendix D: Non Functional Requirements
- Appendix E: Glossary
- Appendix F: Instructions for Manual Testing
- F.1. Launch
- F.2. Playing tracks
- F.3. Pausing
- F.4. Unpausing
- F.5. Stopping
- F.6. Viewing duration of track
- F.7. Seeking a track
- F.8. Creating a new playlist
- F.9. Searching playlists and tracks
- F.10. Listing playlists and tracks
- F.11. Adding tracks to playlist
- F.12. Deleting tracks in a playlist
- F.13. Deleting a playlist
- F.14. Thank you for testing our project! <3
1. Setting up
1.1. Prerequisites
-
JDK
9
or laterJDK 10
on Windows will fail to run tests in headless mode due to a JavaFX bug. Windows developers are highly recommended to use JDK9
. -
IntelliJ IDE
IntelliJ by default has Gradle and JavaFx plugins installed.
Do not disable them. If you have disabled them, go toFile
>Settings
>Plugins
to re-enable them.
1.2. Setting up the project in your computer
-
Fork this repo, and clone the fork to your computer
-
Open IntelliJ (if you are not in the welcome screen, click
File
>Close Project
to close the existing project dialog first) -
Set up the correct JDK version for Gradle
-
Click
Configure
>Project Defaults
>Project Structure
-
Click
New…
and find the directory of the JDK
-
-
Click
Import Project
-
Locate the
build.gradle
file and select it. ClickOK
-
Click
Open as Project
-
Click
OK
to accept the default settings -
Open a console and run the command
gradlew processResources
(Mac/Linux:./gradlew processResources
). It should finish with theBUILD SUCCESSFUL
message.
This will generate all resources required by the application and tests. -
Open
MainWindow.java
and check for any code errors-
Due to an ongoing issue with some of the newer versions of IntelliJ, code errors may be detected even if the project can be built and run successfully
-
To resolve this, place your cursor over any of the code section highlighted in red. Press ALT+ENTER, and select
Add '--add-modules=…' to module compiler options
for each error
-
-
Repeat this for the test folder as well (e.g. check
HelpWindowTest.java
for code errors, and if so, resolve it the same way)
1.3. Verifying the setup
-
Run the
seedu.jxmusic.MainApp
and try a few commands -
Run the tests to ensure they all pass.
1.4. Configurations to do before writing code
1.4.1. Configuring the coding style
This project follows oss-generic coding standards. IntelliJ’s default style is mostly compliant with ours but it uses a different import order from ours. To rectify,
-
Go to
File
>Settings…
(Windows/Linux), orIntelliJ IDEA
>Preferences…
(macOS) -
Select
Editor
>Code Style
>Java
-
Click on the
Imports
tab to set the order-
For
Class count to use import with '*'
andNames count to use static import with '*'
: Set to999
to prevent IntelliJ from contracting the import statements -
For
Import Layout
: The order isimport static all other imports
,import java.*
,import javax.*
,import org.*
,import com.*
,import all other imports
. Add a<blank line>
between eachimport
-
Optionally, you can follow the UsingCheckstyle.adoc document to configure Intellij to check style-compliance as you write code.
1.4.2. Updating documentation to match your fork
After forking the repo, the documentation will still have the CS2103-AY1819S1-T13-3 branding and refer to the CS2103-AY1819S1-T13-3/main
repo.
If you plan to develop this fork as a separate product (i.e. instead of contributing to CS2103-AY1819S1-T13-3/main
), you should do the following:
-
Configure the site-wide documentation settings in
build.gradle
, such as thesite-name
, to suit your own project. -
Replace the URL in the attribute
repoURL
inDeveloperGuide.adoc
andUserGuide.adoc
with the URL of your fork.
1.4.3. Setting up CI
Set up Travis to perform Continuous Integration (CI) for your fork. See UsingTravis.adoc to learn how to set it up.
After setting up Travis, you can optionally set up coverage reporting for your team fork (see UsingCoveralls.adoc).
Coverage reporting could be useful for a team repository that hosts the final version but it is not that useful for your personal fork. |
Optionally, you can set up AppVeyor as a second CI (see UsingAppVeyor.adoc).
Having both Travis and AppVeyor ensures your App works on both Unix-based platforms and Windows-based platforms (Travis is Unix-based and AppVeyor is Windows-based) |
1.4.4. Getting started with coding
When you are ready to start coding,
-
Get some sense of the overall design by reading Section 2.1, “Architecture”.
-
Take a look at [GetStartedProgramming].
2. Design
2.1. Architecture
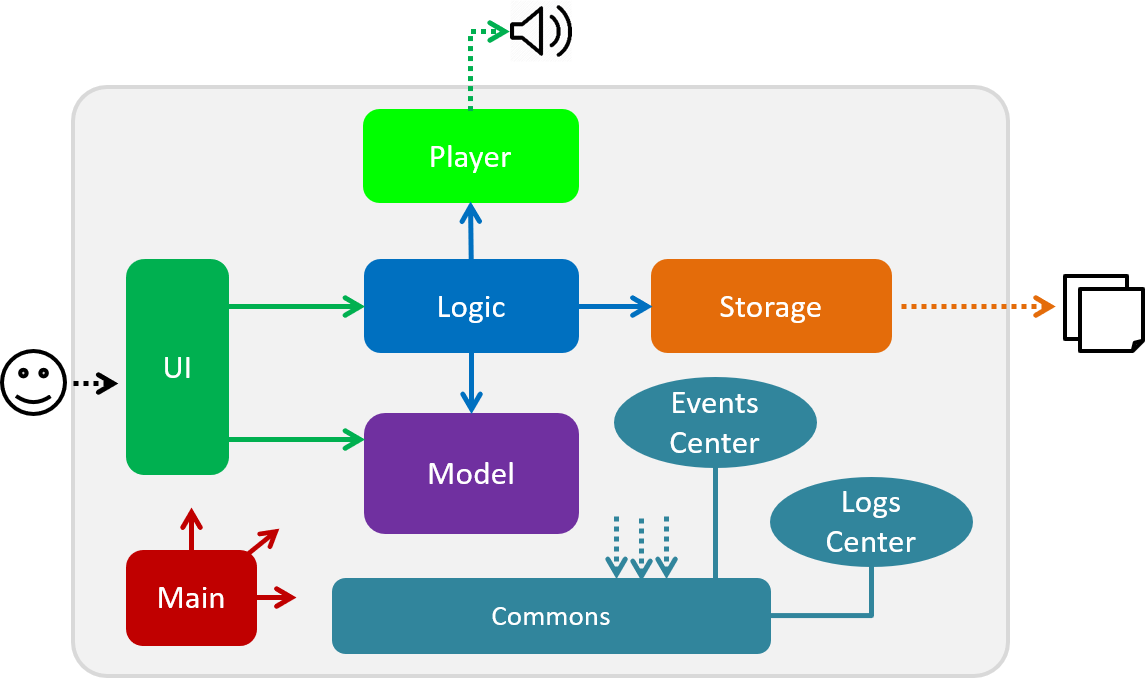
The Architecture Diagram given above explains the high-level design of the App. Given below is a quick overview of each component.
The .pptx files used to create diagrams in this document can be found in the diagrams folder. To update a diagram, modify the diagram in the pptx file, select the objects of the diagram, and choose Save as picture .
|
Main
has only one class called MainApp
. It is responsible for,
-
At app launch: Initializes the components in the correct sequence, and connects them up with each other.
-
At shut down: Shuts down the components and invokes cleanup method where necessary.
Commons
represents a collection of classes used by multiple other components. Two of those classes play important roles at the architecture level.
-
EventsCenter
: This class (written using Google’s Event Bus library) is used by components to communicate with other components using events (i.e. a form of Event Driven design) -
LogsCenter
: Used by many classes to write log messages to the App’s log file.
The rest of the App consists of four components.
Each of the four components
-
Defines its API in an
interface
with the same name as the Component. -
Exposes its functionality using a
{Component Name}Manager
class.
For example, the Logic
component (see the class diagram given below) defines it’s API in the Logic.java
interface and exposes its functionality using the LogicManager.java
class.
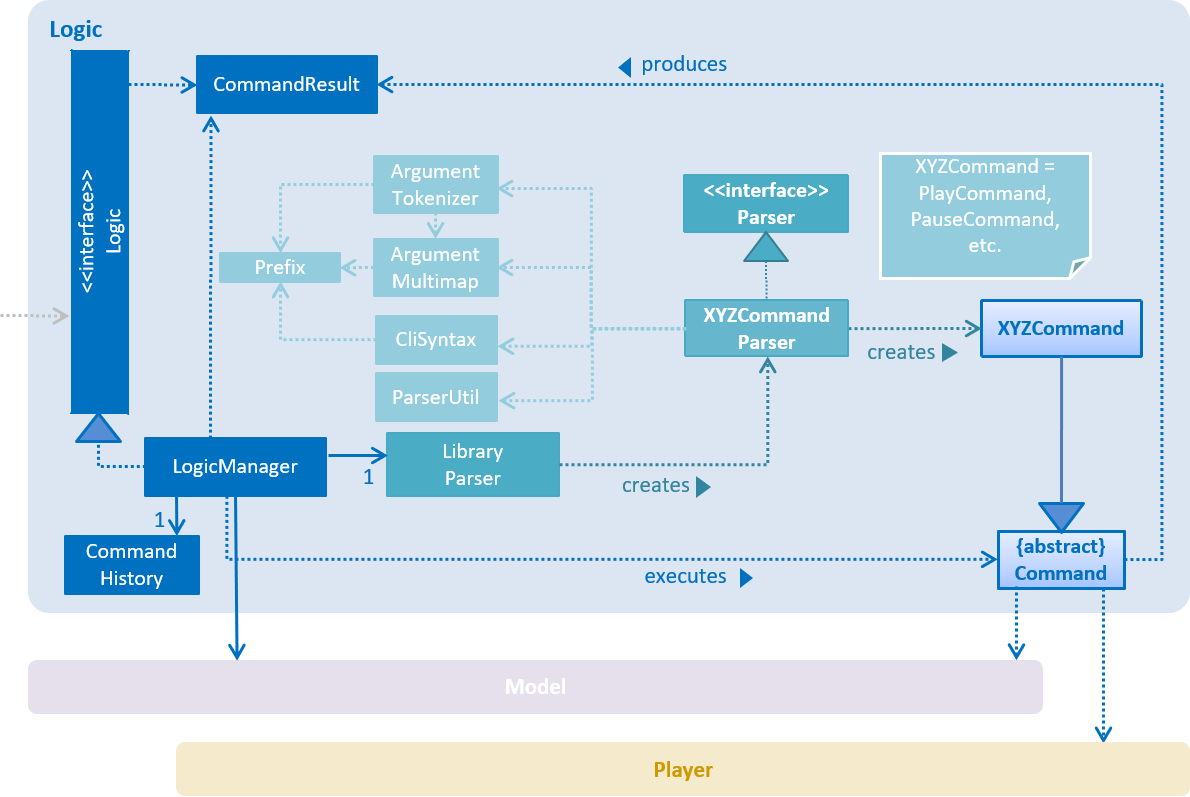
Events-Driven nature of the design
The Sequence Diagram below shows how the components interact for the scenario where the user issues the command playlist del 1
.
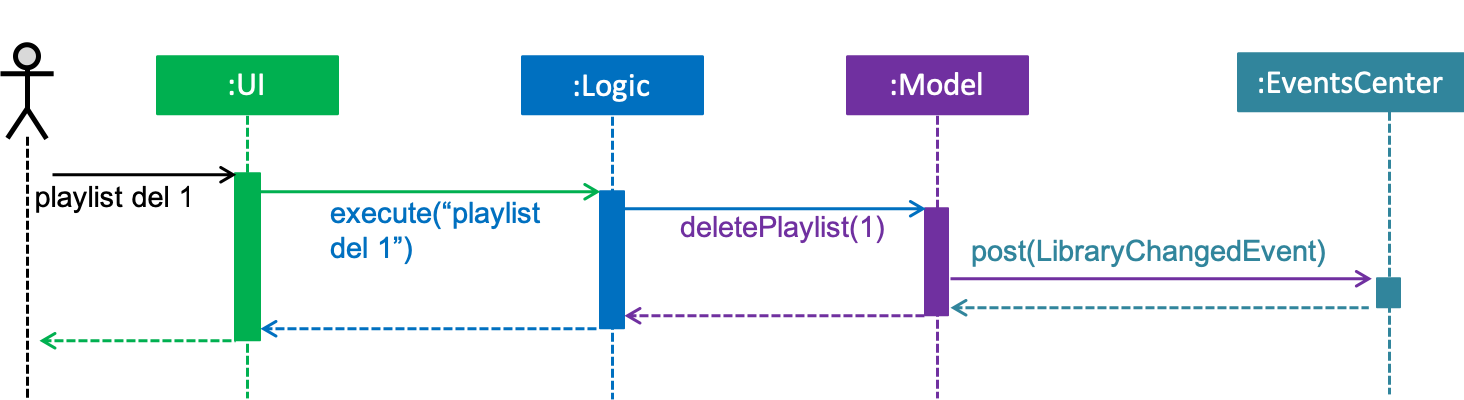
playlist del 1
command (part 1)
Note how the Model simply raises a LibraryChangedEvent when the library data are changed, instead of asking the Storage to save the updates to the hard disk.
|
The diagram below shows how the EventsCenter
reacts to that event, which eventually results in the updates being saved to the hard disk and the status bar of the UI being updated to reflect the 'Last Updated' time.
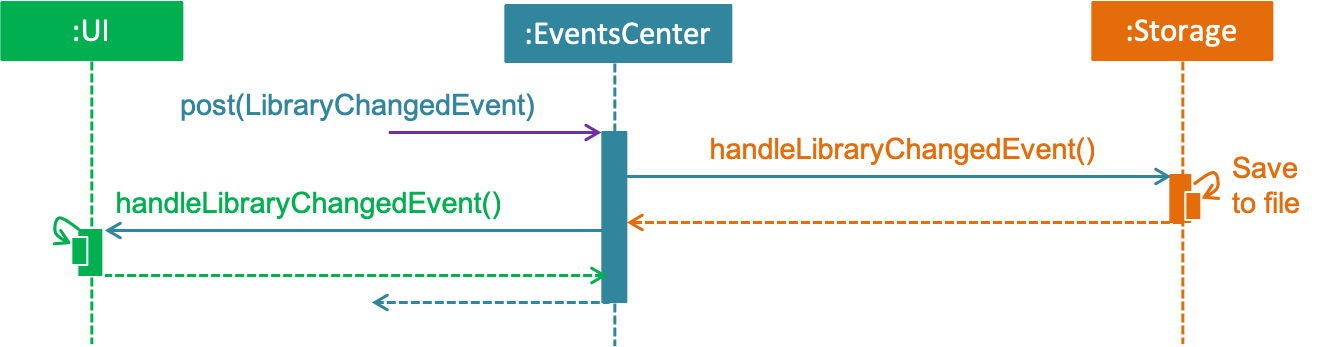
playlist del 1
command (part 2)
Note how the event is propagated through the EventsCenter to the Storage and UI without Model having to be coupled to either of them. This is an example of how this Event Driven approach helps us reduce direct coupling between components.
|
The sections below give more details of each component.
2.2. UI component
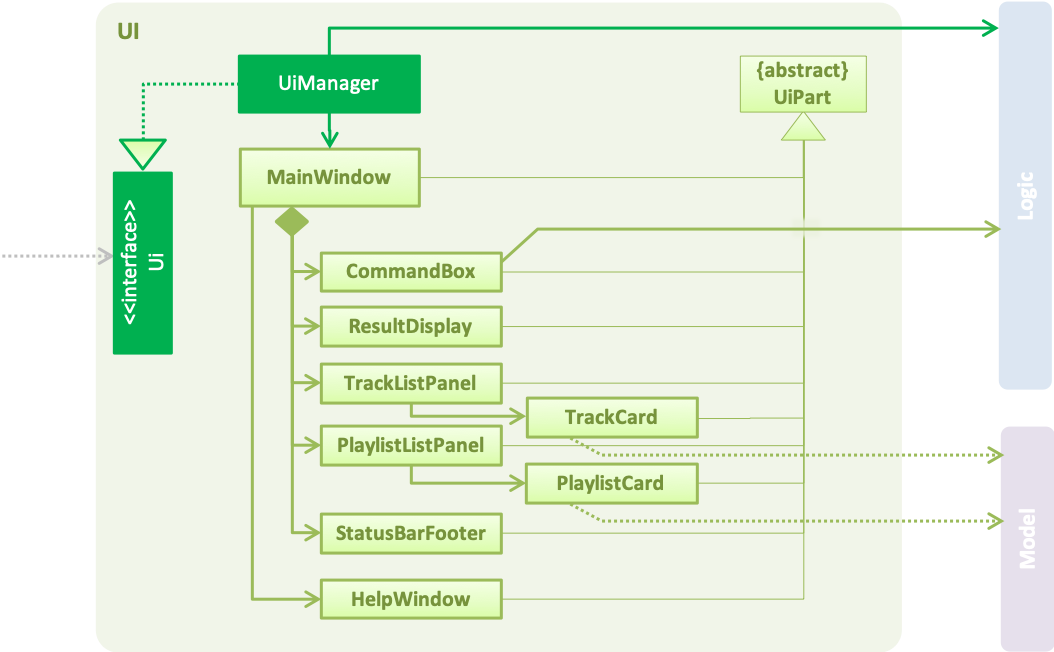
API : Ui.java
The UI consists of a MainWindow
that is made up of parts e.g.CommandBox
, ResultDisplay
, PersonListPanel
, StatusBarFooter
, TrackListPanel
etc. All these, including the MainWindow
, inherit from the abstract UiPart
class.
The UI
component uses JavaFx UI framework. The layout of these UI parts are defined in matching .fxml
files that are in the src/main/resources/view
folder. For example, the layout of the MainWindow
is specified in MainWindow.fxml
The UI
component,
-
Executes user commands using the
Logic
component. -
Binds itself to some data in the
Model
so that the UI can auto-update when data in theModel
change. -
Responds to events raised from various parts of the App and updates the UI accordingly.
2.3. Logic component
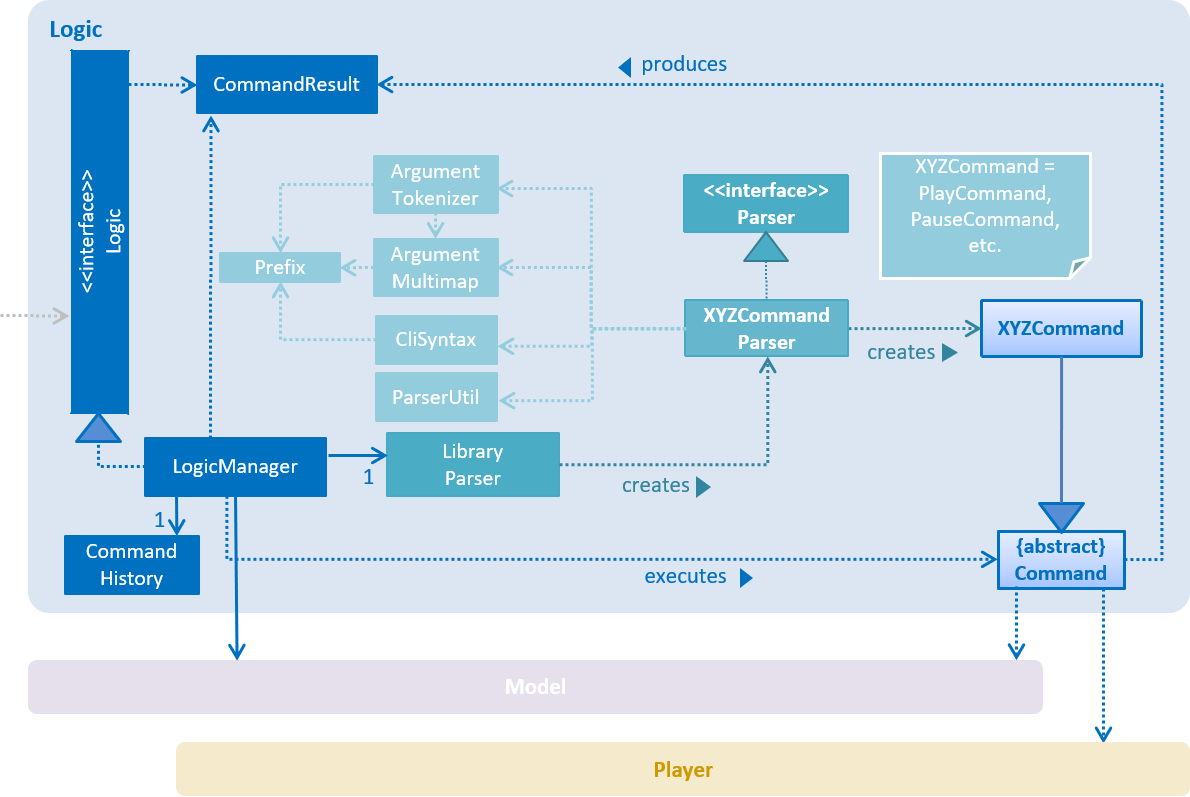
API :
Logic.java
-
Logic
uses theLibraryParser
class to parse the user command. -
This results in a
Command
object which is executed by theLogicManager
. -
The command execution can affect the
Model
(e.g. adding a playlist) and/or raise events. -
The result of the command execution is encapsulated as a
CommandResult
object which is passed back to theUi
.
Given below is the Sequence Diagram for interactions within the Logic
component for the execute("playlist del 1")
API call.
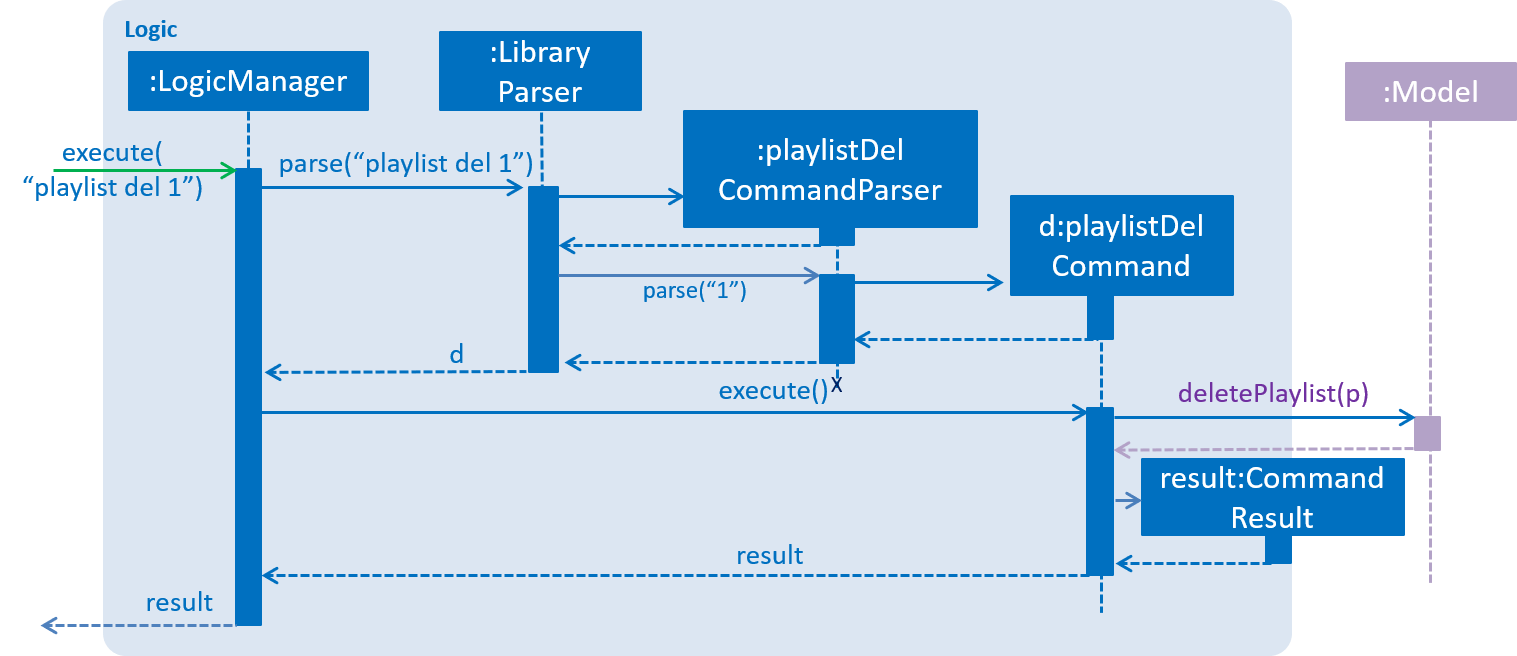
playlist del 1
Command2.4. Model component
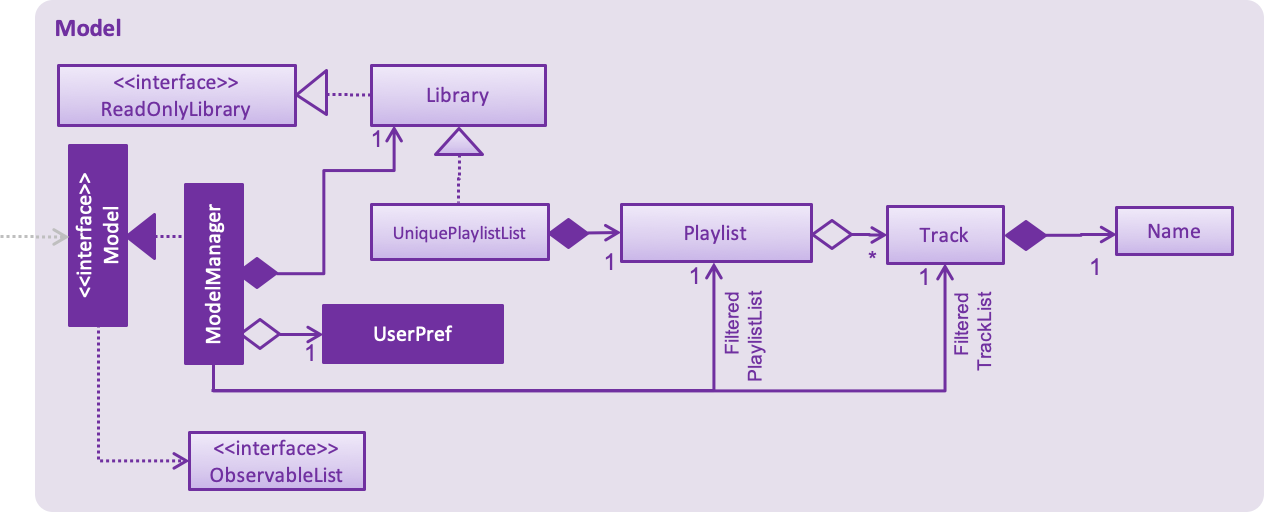
API : Model.java
The Model
,
-
stores a
UserPref
object that represents the user’s preferences. -
stores the Library data.
-
exposes an unmodifiable
ObservableList<Track>
andObservableList<Playlist>
that can be 'observed' e.g. the UI can be bound to this list so that the UI automatically updates when the data in the list change. -
does not depend on any of the other three components.
2.5. Storage component
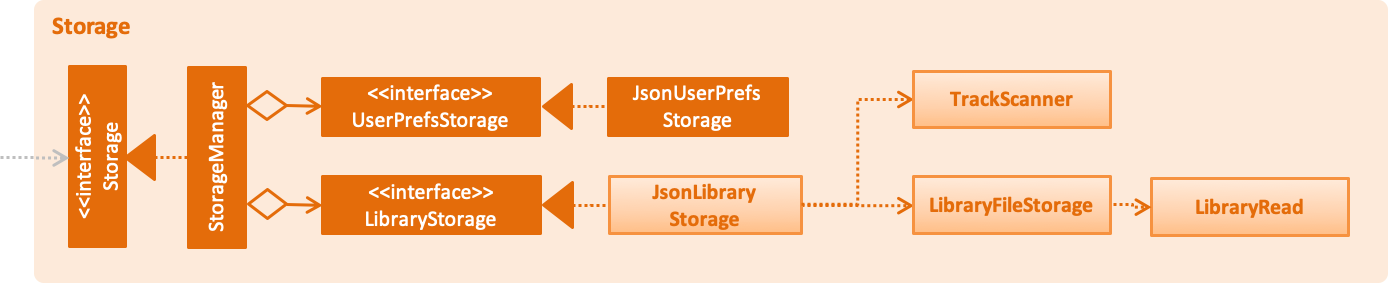
API : Storage.java
The Storage
component,
-
can save
UserPref
objects in json format and read it back. -
can save the library data in json format and read it back.
2.6. Player component
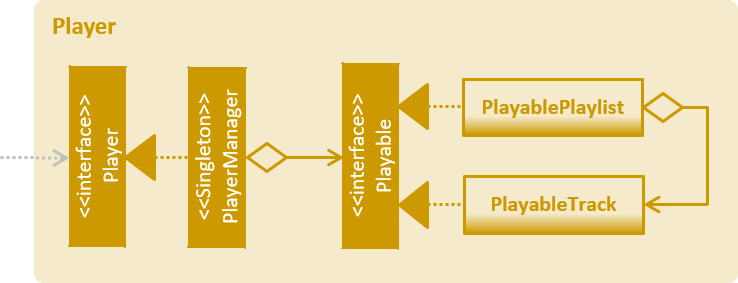
API : Player.java
The Player
component,
-
interfaces with JavaFX Media to play sounds
-
handles media control with mp3 files
2.7. Common classes
Classes used by multiple components are in the seedu.jxmusic.commons
package.
3. Implementation
This section describes some noteworthy details on how certain features are implemented.
3.1. Player Component
3.1.1. Current Implementation
The Player component is a new component in addition to the existing 4 other components of AddressBook. It handles all audio related functionalities for some of the Command classes to use. Player mainly interacts with the JavaFX media library that is included in Java, so no third party library is involved.
The job of the Player is basically forwarding requests of media playback controls to the Playable object.
Singleton Player
Player is implemented as a singleton as only one instance of it is required at any time. While singleton brings about undesired implications such as tighter coupling and lower testability, we ensured that Player is only used by the Command classes and no other parts of the code touches Player. As for testability, we discover that JUnit is not compatible for JavaFX media (details in Section 3.1.1.4, “Notable Issues with JavaFX Media”). On the plus side, singleton pattern makes adding dependency very easy which is very helpful as adding dependency into the Logic component of Address Book was tedious.
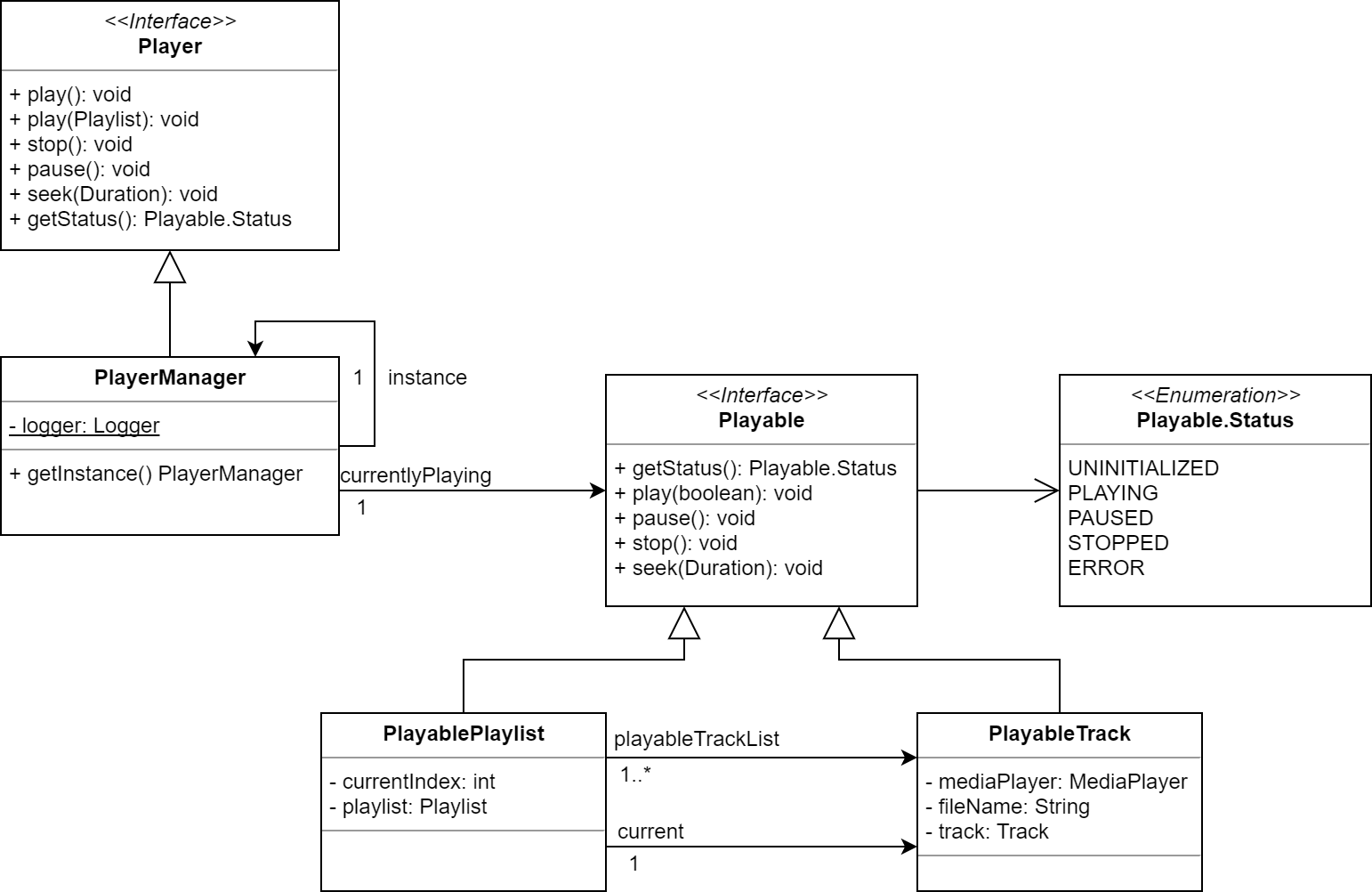
PlayableStatus
PlayableStatus
to represents the state of the player, effectively acts as a layer on top of JavaFX’s MediaPlayer.Status
.
-
UNINITIALIZED
- The initial state when no track or playlist has been played -
PLAYING
- User enters either theplay p/
orplay t/
command -
PAUSED
- User enters thepause
command -
STOPPED
- User enters thestop
command -
ERROR
- Any other states of theMediaPlayer.Status
which JxMusic is not concerned with
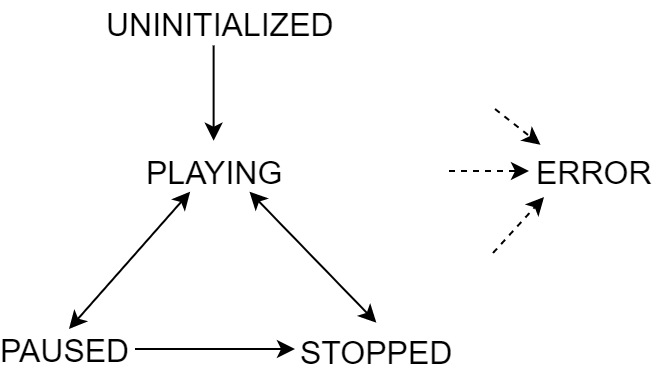
PlayableStatus
state chart diagramSkipped Tests
JUnit and JavaFX MediaPlayer does not work well together. Running any test that constructs a MediaPlayer object (ie new MediaPlayer
) will throw IllegalStateException: Toolkit not initialized
. In order to resolve the exception, it requires calling Platform.startup()
before new MediaPlayer
is called. Even so, the tests will not work on Travis nor Appveyor, throwing MediaException: Cannot create player!
thus failing the builds. It is suspected to be due to incompatibility or lack of support for JavaFX Media on their test servers since JavaFX Media has its own dependencies (at the bottom of link).
Therefore, any test that depends on MediaPlayer are skipped by using Assume.assumeNoException(mediaException)
.
Notable Issues with JavaFX Media
-
JavaFX Media does not work for mp3 files that has photoshopped album art.
-
This issue has been reported as a confirmed Java bug.
-
-
Playing a track on MacOS requires setting the MediaPlayer current time to 0 before calling
play()
as it jumps to the end of media for no reason. Whereas on Windows, callingMediaPlayer.play
works.-
This issue is addressed at #35.
-
3.1.2. Design Considerations
Aspect: Choice of media player library
-
Alternative 1 (current choice): Use JavaFX Media API
-
Pros:
-
No additional dependency (at least on common operating systems)
-
-
Cons:
-
-
Alternative 2: Use 3rd party mp3 player library such as JLayer
-
Pros:
-
Possibly less issues than JavaFX Media.
-
-
Cons:
-
Additional dependency
-
Existing mp3 libraries for java are old and badly documented.
-
-
3.2. ReadLibrary
3.2.1. Current Implementation
The readLibrary
is a part of JsonLibraryStorage
. It reads the Json file as well as the library folder when initialising the library
class. It calls the following operations:
-
JsonFileStorage.loadDataFromFile(filepath)
- deserialize the Json file and createlibrary
class. -
Trackscanner.scan(libraryDir)
- scan through the library folder and fill all the valid mp3 files into a set of tracks.
No matter whether the Json file exists, trackscanner
will run first to make sure the library
class contains the tracks set. Then if the file path exists, it will deserialize the json file to initialize library’s playlist information. The deserializer and serializer are programmed with Gson
library.
The trackscanner
navigates to the library folder and extracts the mp3 files and add them to the set of tracks. However there are cases that the user does not have a library folder in the same directory as the app. To ensure the tracks contained inside the playlist to be valid and navigable, we put a library folder containing default tracks inside the resources folder.
Hence, the program first extracts the library folder from the jar file and copy it to the directory. There are two methods that the app copies library to the directory:
-
Case 1: If the MainApp is run using jar file,
trackscanner
will internally run the unzip command to unzip the jar file. (Warning: This only works if the jar file is opened using Terminal); -
Case 2: If the MainApp is run using IDE,
trackscanner
will copy the library folder from resources to the directory;
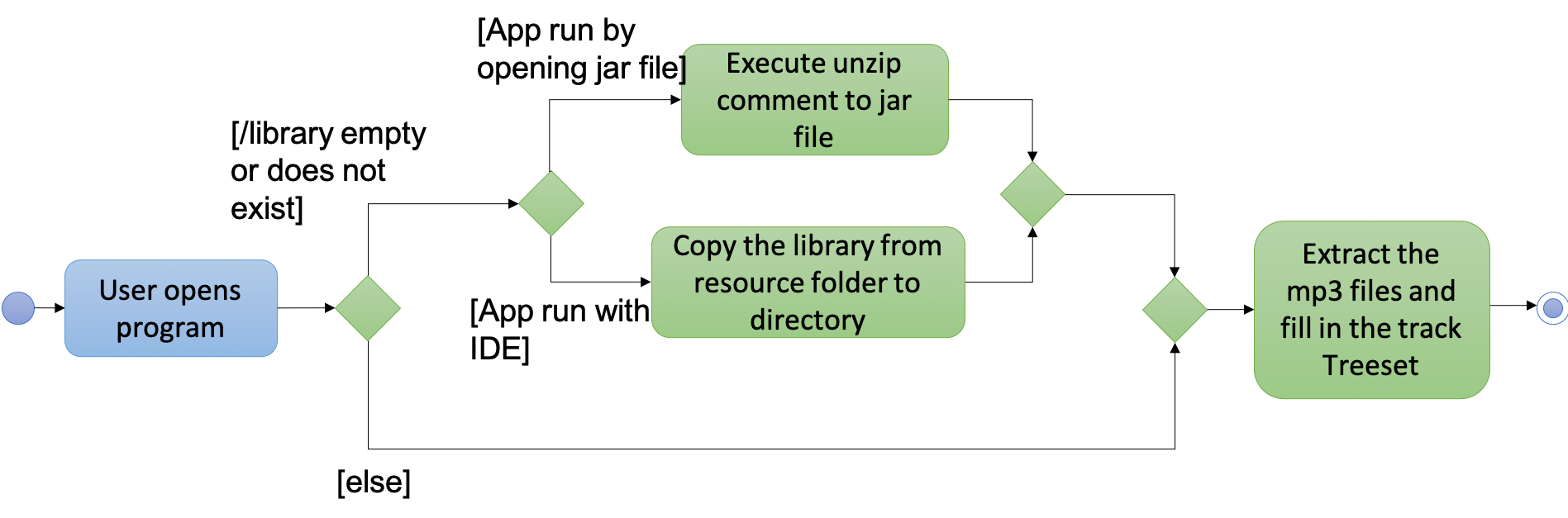
3.2.2. Design Considerations
Aspect: Choice of media player library
-
Alternative 1 (current choice): Read the Json file from data folder and scan the tracks from library folder at the same time.
-
Pros:
-
Enables the library to initialise with all the available tracks in library folder
-
-
Cons:
-
The track treeset in the library class will not update again after initialisation.
-
-
-
Alternative 2: Do not scan the tracks in
readLibrary
, scan the library each time the user search for tracks in the library folder.-
Pros:
-
Lead to the files’ latest status inside the library folder
-
-
3.2.3. Future Enhancements
When the user double clicks the jar file it cannot run because the unzipping of jar file is done using process.exec
, which requires the user to run the jar file using terminal. This needs to be addressed in future development.
3.3. Track search and Track list
3.3.1. Current Implementation
The tracklist
and tracksearch
commands are implemented to view and filter tracks in the library.
-
Command.TrackListCommand
- list all the tracks inside the library -
Command.TrackSearchCommand
- search the desired tracks from library whose name contains any of the argument keywords.
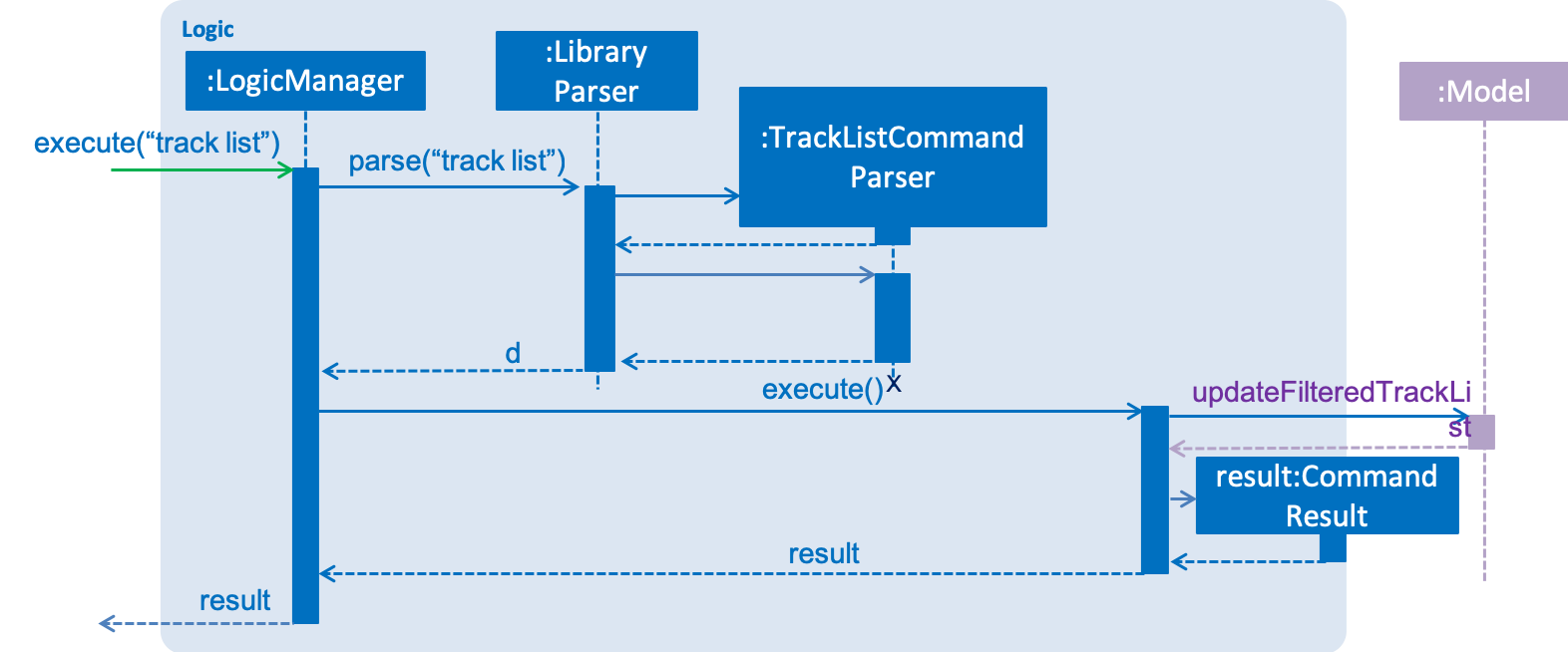
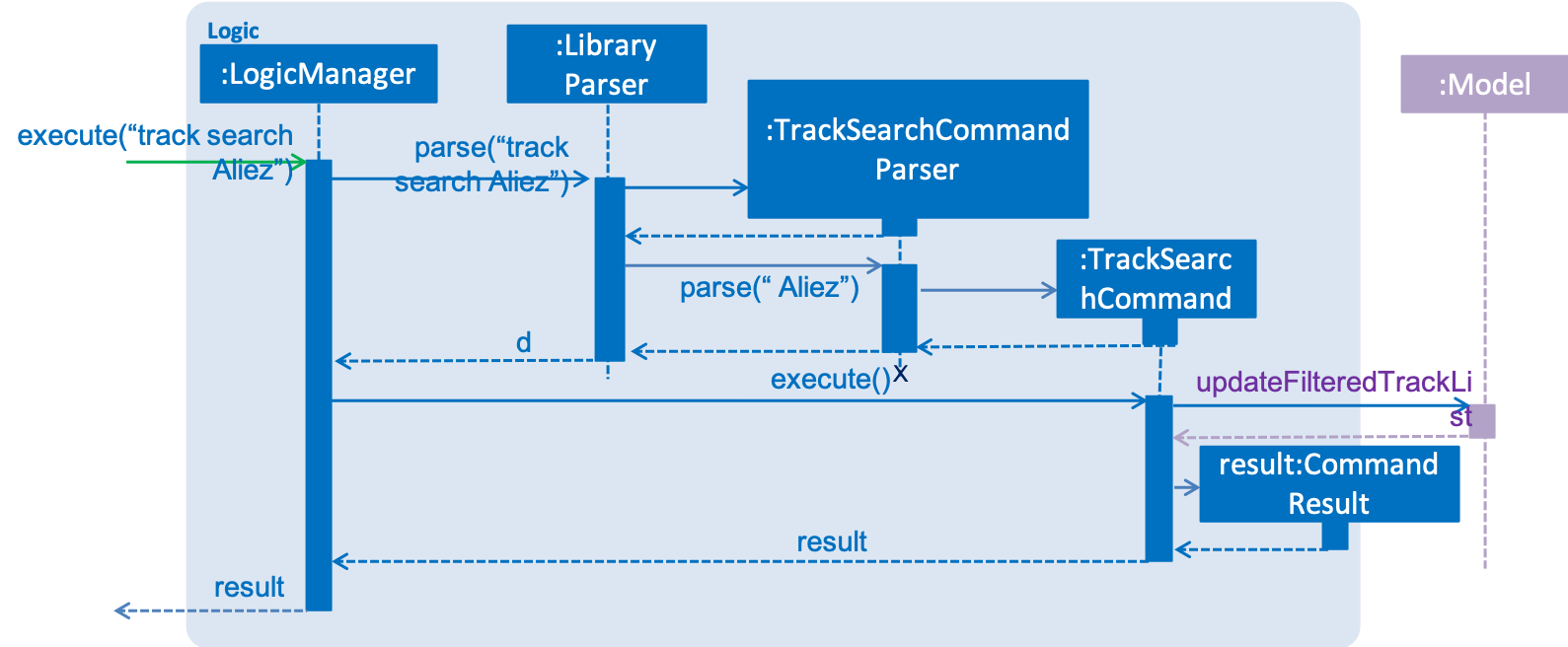
3.3.2. Design Considerations
Aspect: Searching multiple keywords.
-
Alternative 1 (current choice): Combines each search results by each keywords separated by space.
-
Pros:
-
Find tracks that match with any of the keywords
-
-
Cons:
-
Spaces are not included in the keywords.
-
-
-
Alternative 2: consider words as one part of the desired track and show results of the search.
-
Pros:
-
Show more precise results
-
-
Aspect: Tracks listed display
-
Alternative 1 (current choice): List the tracks in a new panel separate from playlist panel.
-
Pros:
-
Tracks are more clearly shown.
-
-
-
Alternative 2: Show the tracks in the message box
-
Pros:
-
Easier to implement.
-
-
Cons:
-
Takes up the message box and hence the success message or failing message cannot be seen.
-
-
3.3.3. Future Enhancements
Future development can combine the two alternatives so that the user can search with keywords including spaces when they intend to.
3.4. Track Adding and Removing Feature
Simple-to-use track management commands that add tracks to a specified playlist.
3.4.1. Current Implementation
When managing a playlist, you can customise it by adding and removing tracks from it.
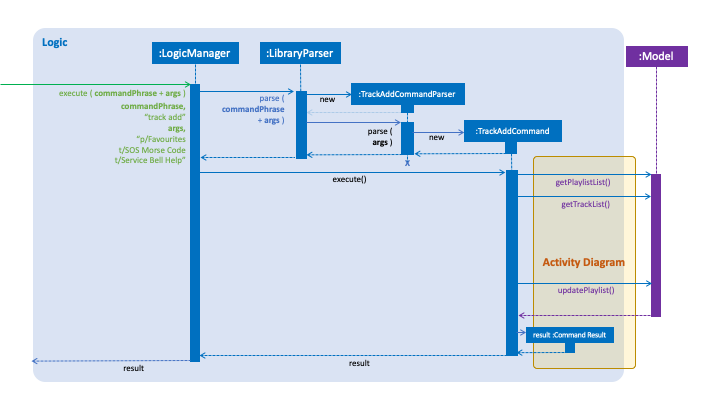
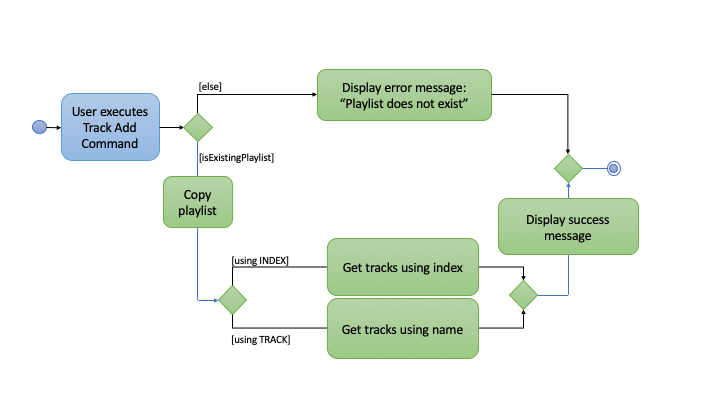
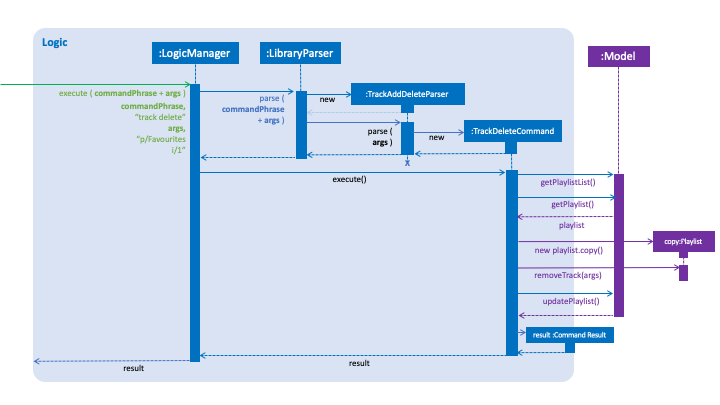
3.4.2. Design Considerations
To add new tracks to a playlist can be a very mechanical task, hence the ease of allowing for adding multiple entries helps to ease the need for repetition. It also makes sense for the inclusion of adding tracks by referring to its index on the panel.
Hence, there are two ways that tracks can be added. Firstly, with track name. Secondly, with its index from filteredTrackList panel.
The omission of repeating the index prefix is also added to reduce typing required to perform deletion. Having to type the prefix with every index means including up to 200% more typing per index to add.
Deleting tracks does not support deletion in multiples, and it omits the need for index prefix.
While tracks can be added by name, it makes sense to allow the user to make case-insensitive references to tracks. This helps to reduce the need to follow case convention for track names.
3.4.3. Future Enhancements
Deleting multiple tracks could be implemented as more advanced features are added.
Adding multiple tracks by substrings can be implemented to facilitate quick modification of playlists.
3.5. Playback Feature
Four functionalities are implemented to achieve the playback task in JxMusic Player. play
enables users to play musics. pause
allows the break off of playing and stop
terminates playing. seek
functionality achieves the requirement of seeking the playing to a certain time point. To aid the use of seek
, duration
command is implemented to let users get the duration of track.
3.5.1. Play
PlayCommand caters for commands having the word play
in it, in particular, play
, play p/
and play t/
commands. It consists of 5 modes:
-
Continue from pause -
play
-
Default playlist -
play p/
-
Specific playlist -
play p/<playlist name>
-
Default track -
play t/
-
Specific track -
play t/<track name>
These modes are determined by the different PlayCommand constructors called by the PlayCommandParser after it parses the user input, as shown in the activity diagram below:
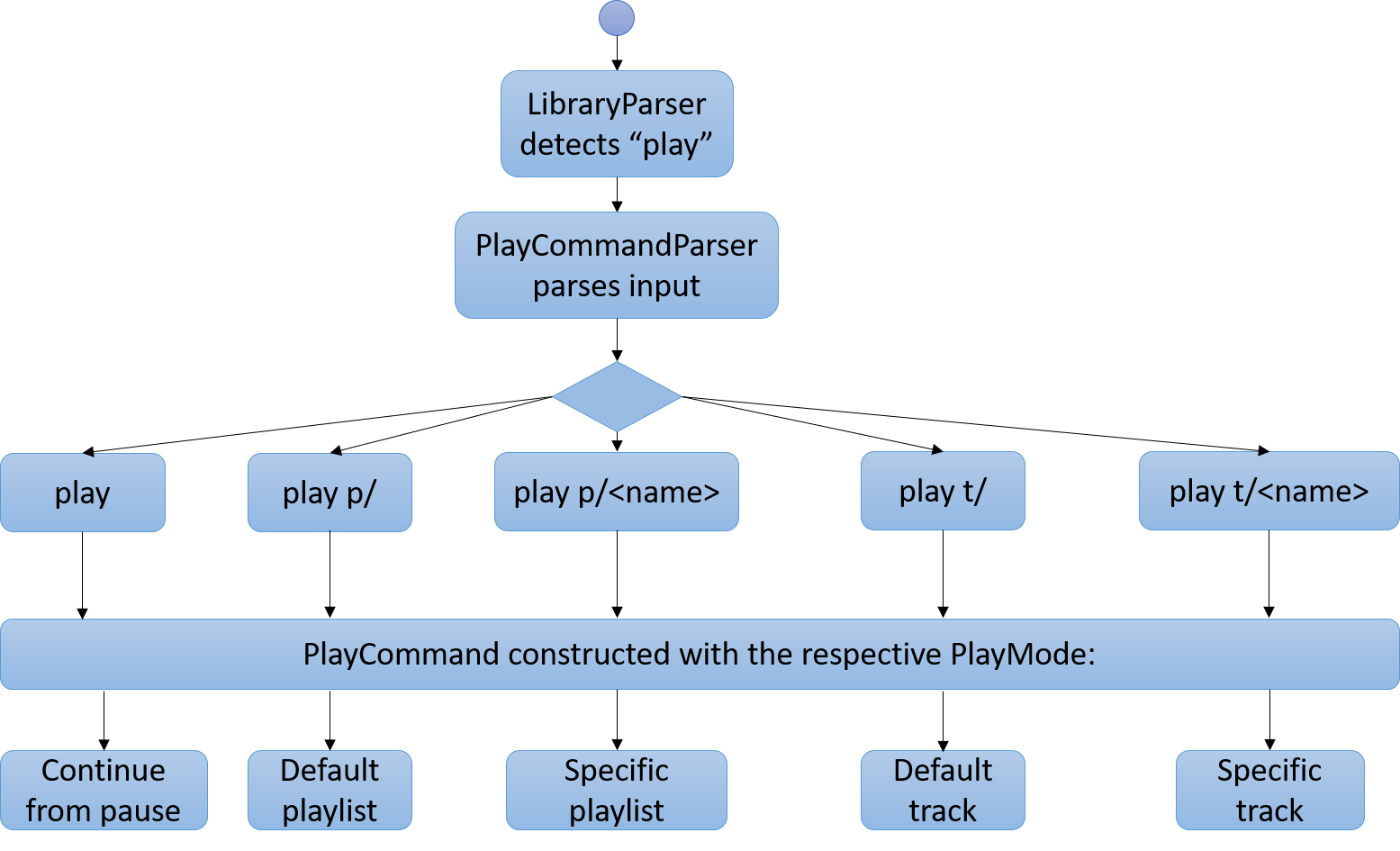
Playing a playlist constructs a PlayablePlaylist which is essentially playing a list of PlayableTrack. The currently playing track is pointed by the currentIndex
.
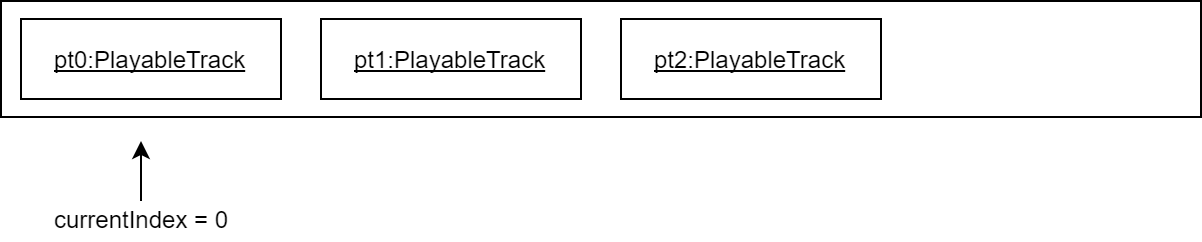
When a track finishes playing, the next track is played.
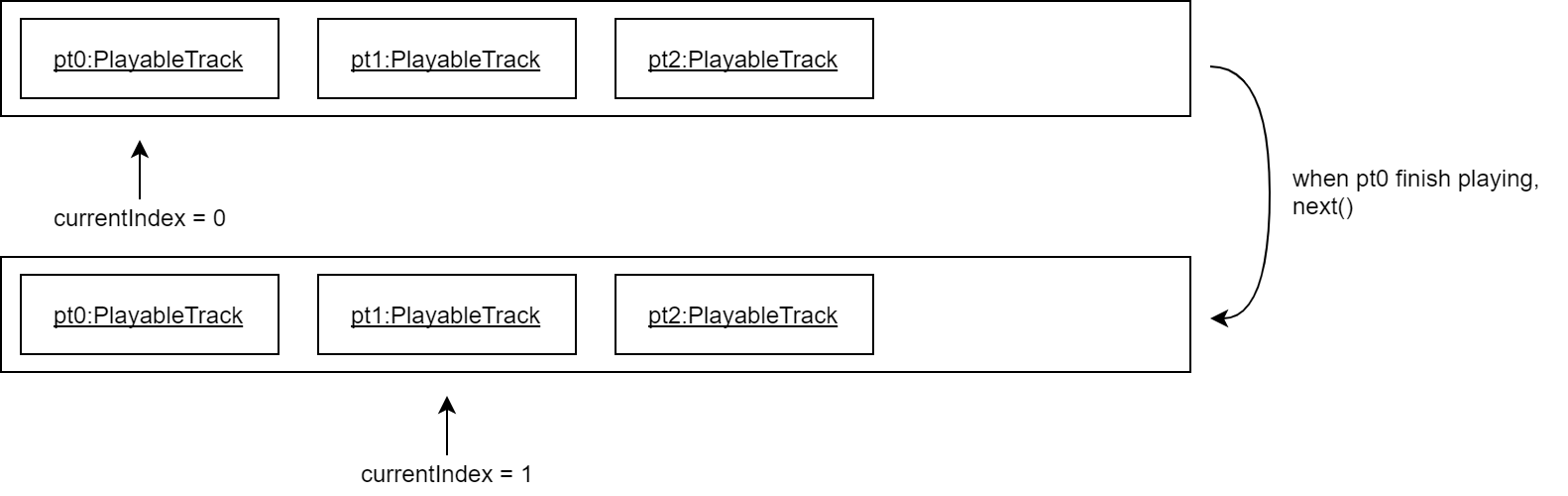
Future enhancement: implement prev()
for playing the previous track.
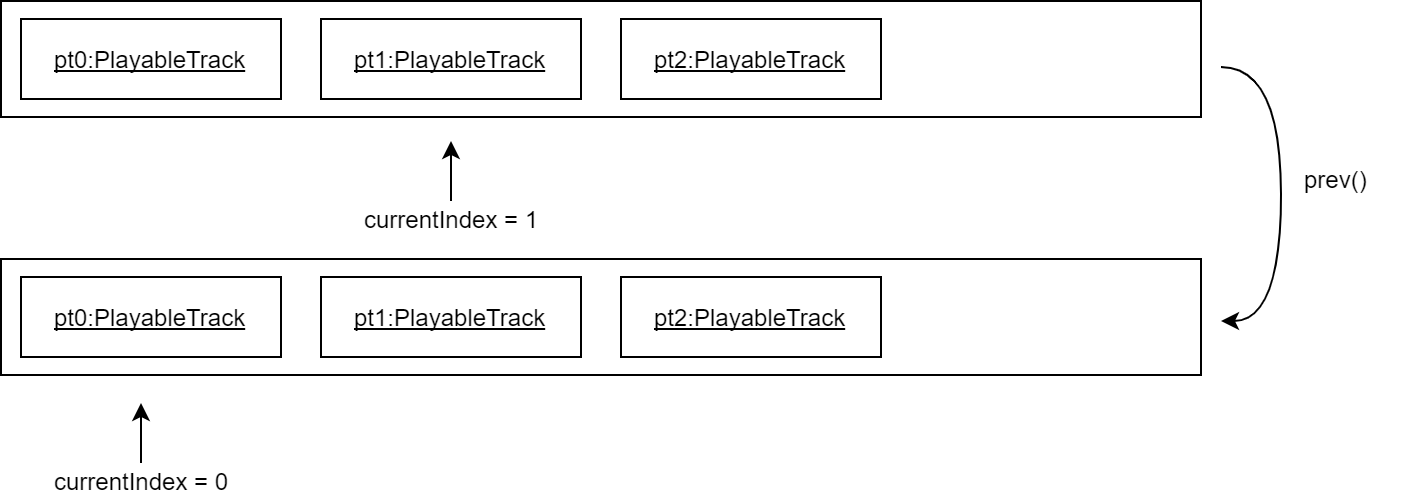
Aspect: Initialization stage of PlayablePlaylist
-
Alternative 1 (current choice): Constructs a list of PlayableTrack
-
Pros:
-
Better performance when switching tracks
-
Easier implementation
-
-
Cons:
-
Does not cater for playlist changes when it is playing (ie adding/deleting a track while the playlist is playing)
-
-
-
Alternative 2: Constructs PlayableTrack only when it is to be played
-
Pros:
-
Caters for playlist changes
-
-
Cons:
-
Slightly worse performance
-
More complex implementation
-
-
3.5.2. Pause
PauseCommand
is implemented to realize the pausing a playing entity.
The playing could be resumed after pause and it will play from the time point at which the playing entity is paused.
Ultimately, pause()
method in javafx.scene.media.MediaPlayer
is being called to achieve the performance.
3.5.3. Stop
StopCommand
is implemented to achieve the termination of playing of a playing entity.
The playing could not be resumed after stop
, but the entity being played is remembered and play
command
after stop
will result in the playing of the entity from the beginning.
Ultimately, stop()
method in javafx.scene.media.MediaPlayer
is being called to achieve the performance.
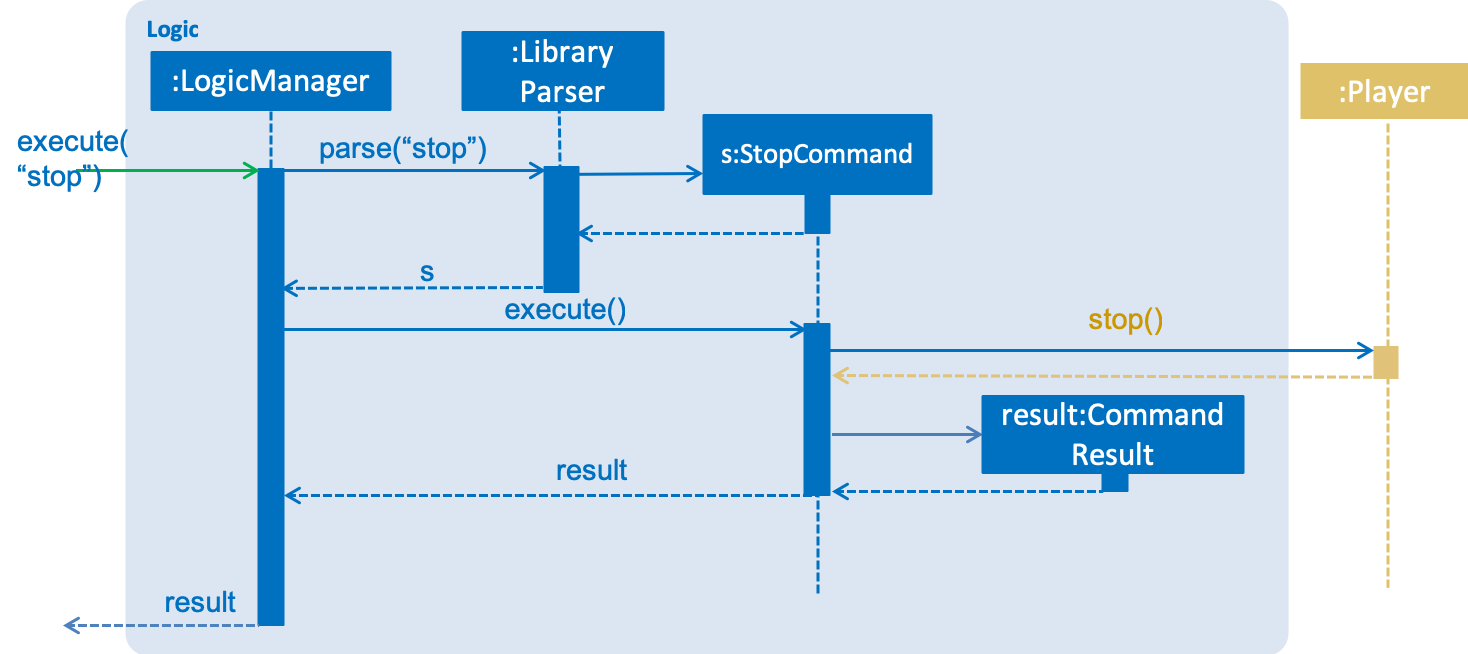
stop
command3.5.4. Seek
Current Implementation
The seek
functionality enables user to seek the play of a track to a certain time point.
This feature is achieved by the implementation of SeekCommand
in logic class.
To perform user’s instruction, SeekCommandParser
is also being implemented. Ultimately, seek(Duration seekTime)
method in javafx.scene.media.MediaPlayer
is being called to achieve the performance.
Aspect: How seek behaves when the time point is beyond start/stop(aka. total) time
-
Alternative 1 (current choice): throws exception with error message

seek
-
Pro:
-
User will get notification message if their intention could not be achieved
-
-
Con:
-
Exception will be thrown and the duration information should be made clear to users.
-
Alternative 2: follows the specification of
seek(Duration seekTime)
injavafx.scene.media.MediaPlayer
In the documentation ofjavafx.scene.media.MediaPlayer.seek(Duration seekTime)
, it specifies the following execution results:
If seekTime > stop time, seek to stop time.
If seekTime < start time, seek to start time.
-
-
-
Pro:
-
No extra implementation of the retrieval of duration information needs to be done.
-
-
Con:
-
User will not get notification message if their intention of seeking to a point out of playing time fails.
-
3.5.5. Duration
Current Implementation
DurationCommand
is implemented to enable users to know the duration of current playing track/paused/stopped track.
A message contains the duration of the track will displayed in ResultDisplay
of the MainWindow
.
Aspect: How user get to know the duration of a track
-
Alternative 1 (current choice): implements a command to retrieve the information of duration
-
Pro:
-
User will have the control of the display of the duration information.
-
-
Con:
-
Compare with Alternative 2, it is less user friendly since extra effort is needed for users to get the information of duration.
-
-
-
Alternative 2: displays the duration of tracks in
trackCard
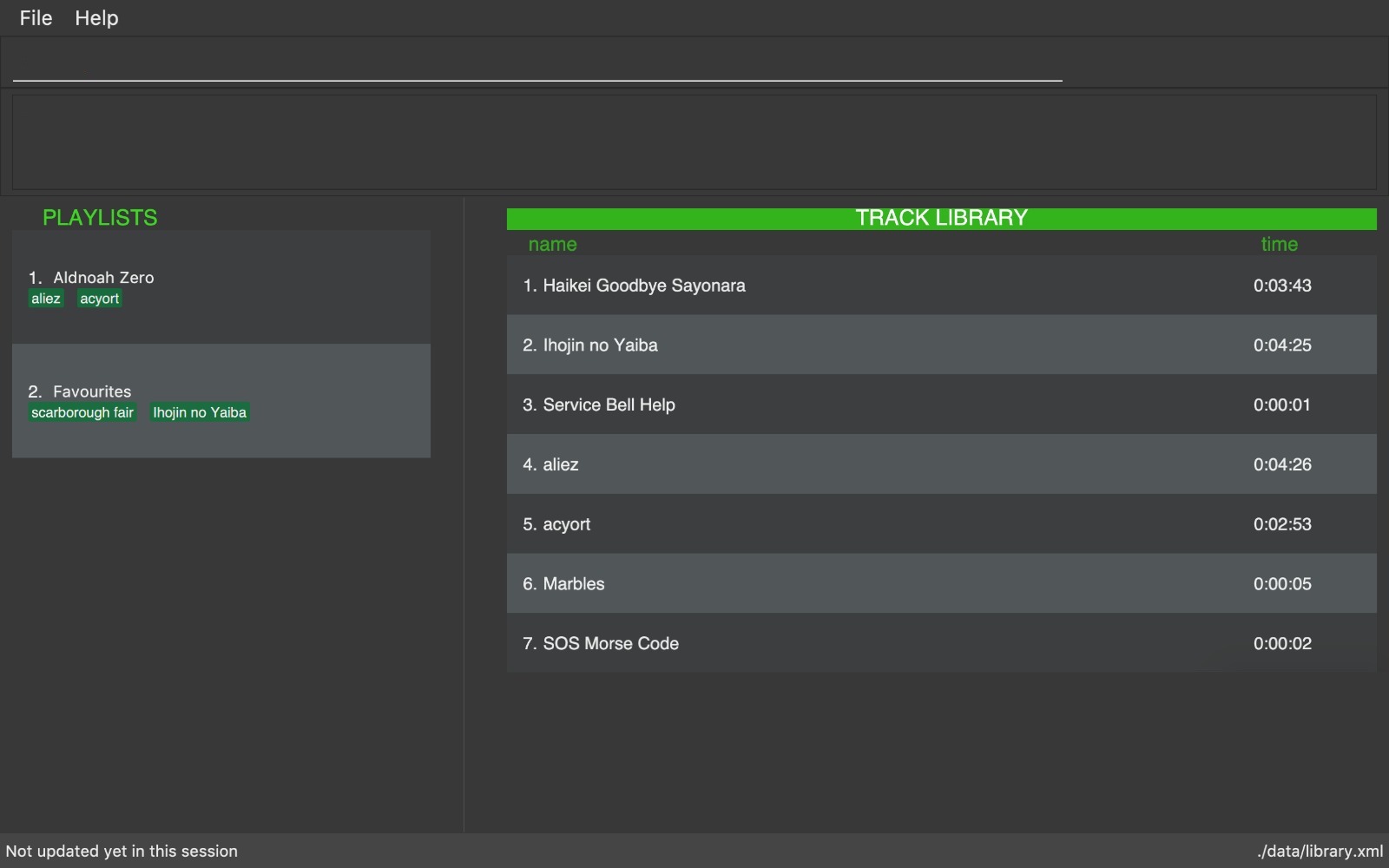
This choice is what we chose at first, but subsequently we find out the some methods could not be tested due to the
backwardness of javafx (Travis and AppVeyor throw com.sun.media.jfxmedia.mediaexception: could not create player!
exception while local tests pass)
-
Pro:
-
It is more convenient and intuitive since no extra effort need to be made to get to know the duration information.
-
-
Con:
-
javafx
feature might be an obstacle when testing.
-
3.6. Playlist Management feature
3.6.1. Current Implementation
Playlist new - Creates a new playlist which is added to the library
Playlist del - Deletes the playlist indexed displayed on the playlist panel from the library
Playlist search - Searches through the library and displays the playlist/s that match the desired String/ sub-String
Playlist list - Lists all playlists within the library
The PlaylistNewCommand creates a new Playlist that is added to the Library object if it does not exists. It’s command phrase pattern is playlist new p/playlist [t/track]…
where playlist
denotes the name of the playlist and track
denotes the name of the tracks.
Additionally, it implements the following operations:
Pattern:
playlist new p/playlist [t/track]…
Example:
playlist new p/Favourites t/Somesong t/Othersong
If no tracks are specified, an empty playlist will be created. Otherwise, the list of tracks will be automatically added into the playlist.
The PlaylistNewCommandParser handles parsing of the user input, specifically for the mandatory playlist name as well as the optional track list.
If there exists a playlist in the library with the same name as the new playlist, it will be rejected from being added into the library as playlist is identified by its name.
The following sequence diagram shows how a new playlist is added:
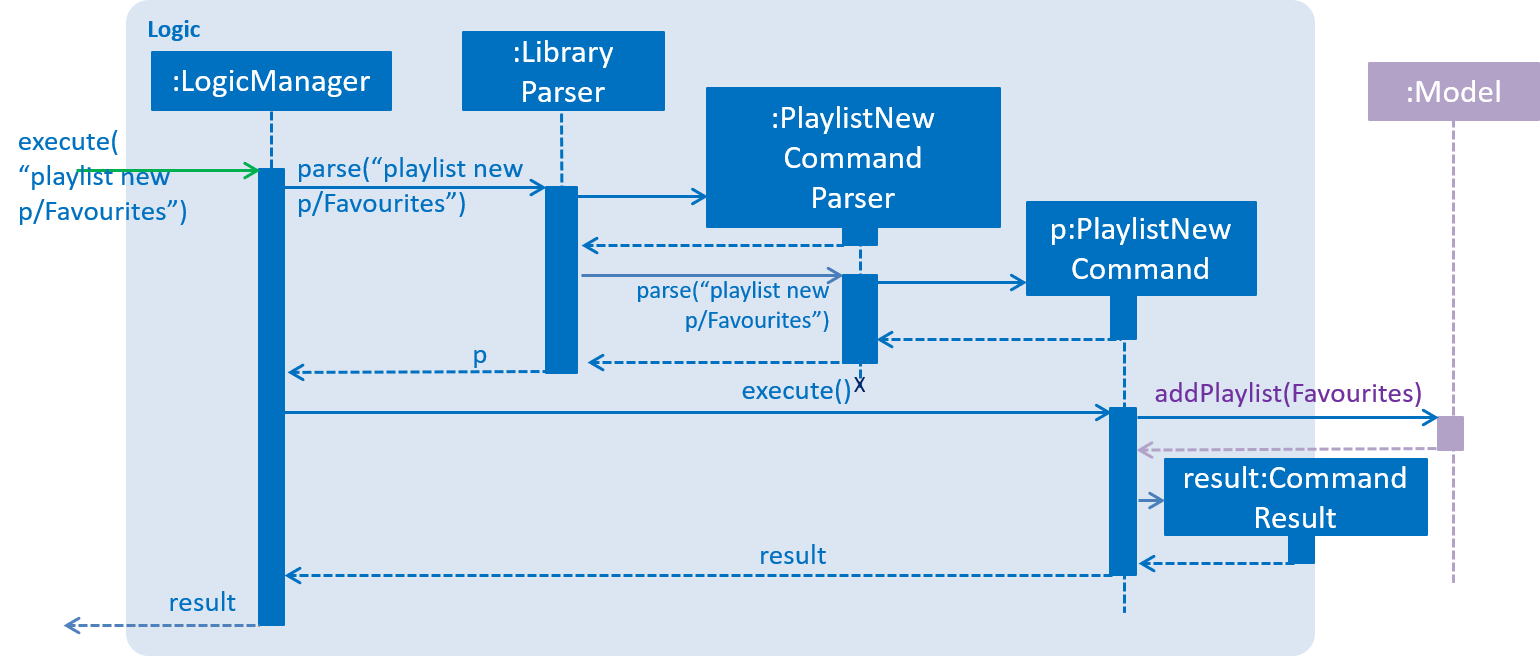
The following activity diagram summarizes what happens when a user executes a new command:
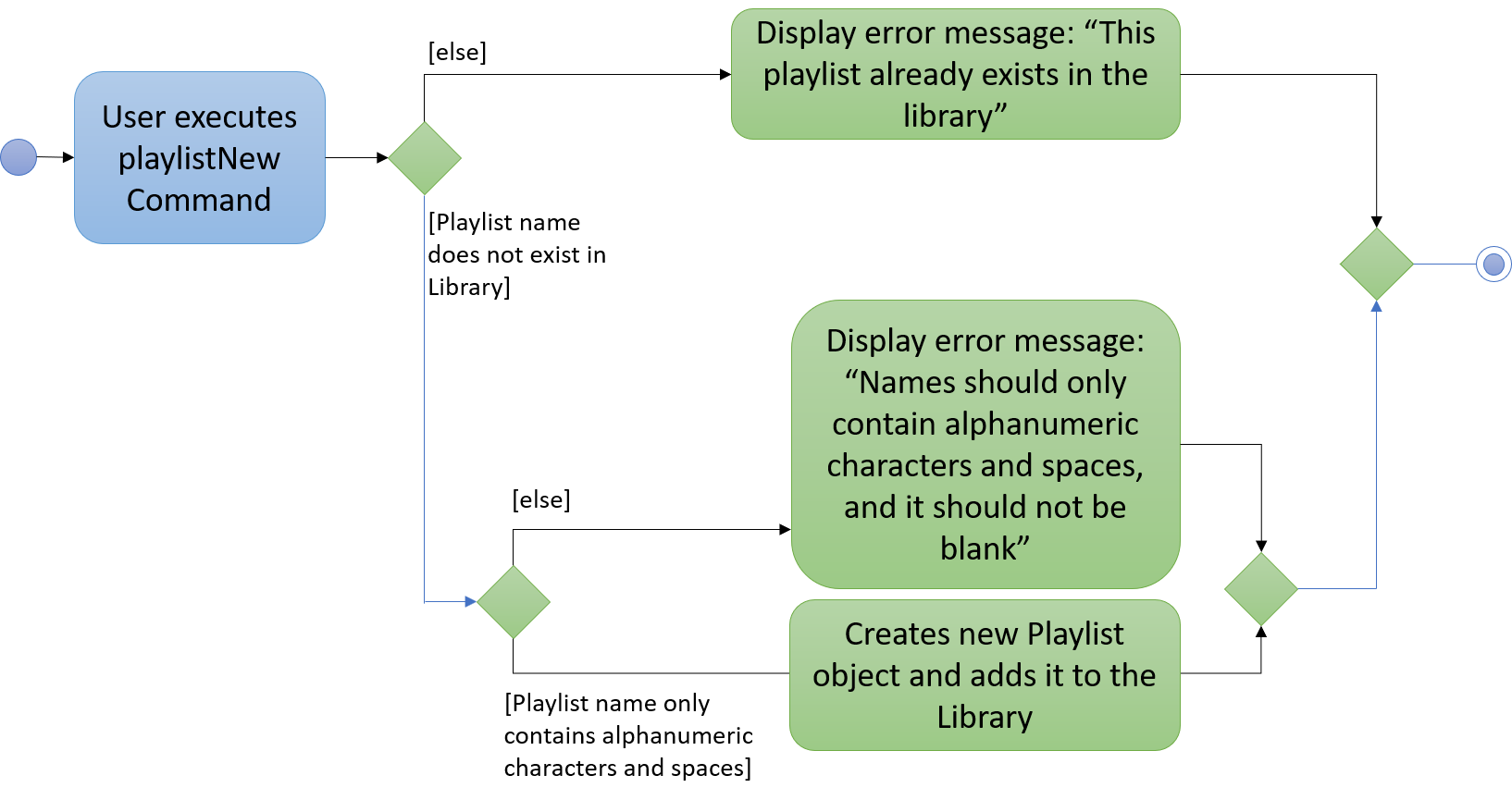
3.6.2. Design Considerations
Aspect: How tracks are added to a new playlist
-
Alternative 1 (current choice): When adding tracks to a new playlist - Identify the tracks by full track name.
-
Pros: Easy to implement and intuitive for the user.
-
Cons: Tracks with long name could take a long time to type.
-
-
Alternative 2: Identify the track via index within the track List panel.
-
Pros: Can be much faster to add tracks
-
Cons: If panel List is large, it could take a long time to find track
-
Aspect: Data structure to support adding a Playlist
-
Alternative 1 (current choice): New playlists are added to the bottom of the Library.
-
Pros: Keeps the most recent / relevant playlists at the highest playlist index number.
-
Cons: Hard to find playlist when Library contains a large amount of playlists.
-
-
Alternative 2: Playlists are displayed in alphabetical order
-
Pros: Easier to find desired playlist within large list.
-
Cons: Harder to find recently added playlists
-
-
Alternative 3: New playlists display at the top of the playlist panel.
-
Pros: Keeps the most recent / relevant playlists at the top and easily accessible.
-
Cons: Has larger compute time cost when list is large
-
3.7. [Proposed] Data Encryption
{Explain here how the data encryption feature will be implemented}
3.8. Logging
We are using java.util.logging
package for logging. The LogsCenter
class is used to manage the logging levels and logging destinations.
-
The logging level can be controlled using the
logLevel
setting in the configuration file (See Section 3.9, “Configuration”) -
The
Logger
for a class can be obtained usingLogsCenter.getLogger(Class)
which will log messages according to the specified logging level -
Currently log messages are output through:
Console
and to a.log
file.
Logging Levels
-
SEVERE
: Critical problem detected which may possibly cause the termination of the application -
WARNING
: Can continue, but with caution -
INFO
: Information showing the noteworthy actions by the App -
FINE
: Details that is not usually noteworthy but may be useful in debugging e.g. print the actual list instead of just its size
3.9. Configuration
Certain properties of the application can be controlled (e.g App name, logging level) through the configuration file (default: config.json
).
4. Documentation
We use asciidoc for writing documentation.
We chose asciidoc over Markdown because asciidoc, although a bit more complex than Markdown, provides more flexibility in formatting. |
4.1. Editing Documentation
See UsingGradle.adoc to learn how to render .adoc
files locally to preview the end result of your edits.
Alternatively, you can download the AsciiDoc plugin for IntelliJ, which allows you to preview the changes you have made to your .adoc
files in real-time.
4.2. Publishing Documentation
See UsingTravis.adoc to learn how to deploy GitHub Pages using Travis.
4.3. Converting Documentation to PDF format
We use Google Chrome for converting documentation to PDF format, as Chrome’s PDF engine preserves hyperlinks used in webpages.
Here are the steps to convert the project documentation files to PDF format.
-
Follow the instructions in UsingGradle.adoc to convert the AsciiDoc files in the
docs/
directory to HTML format. -
Go to your generated HTML files in the
build/docs
folder, right click on them and selectOpen with
→Google Chrome
. -
Within Chrome, click on the
Print
option in Chrome’s menu. -
Set the destination to
Save as PDF
, then clickSave
to save a copy of the file in PDF format. For best results, use the settings indicated in the screenshot below.
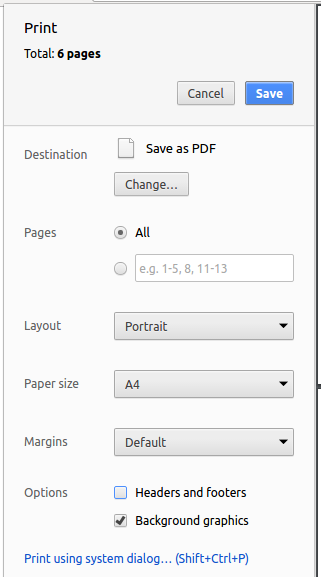
4.4. Site-wide Documentation Settings
The build.gradle
file specifies some project-specific asciidoc attributes which affects how all documentation files within this project are rendered.
Attributes left unset in the build.gradle file will use their default value, if any.
|
Attribute name | Description | Default value |
---|---|---|
|
The name of the website. If set, the name will be displayed near the top of the page. |
not set |
|
URL to the site’s repository on GitHub. Setting this will add a "View on GitHub" link in the navigation bar. |
not set |
|
Define this attribute if the project is an official SE-EDU project. This will render the SE-EDU navigation bar at the top of the page, and add some SE-EDU-specific navigation items. |
not set |
4.5. Per-file Documentation Settings
Each .adoc
file may also specify some file-specific asciidoc attributes which affects how the file is rendered.
Asciidoctor’s built-in attributes may be specified and used as well.
Attributes left unset in .adoc files will use their default value, if any.
|
Attribute name | Description | Default value |
---|---|---|
|
Site section that the document belongs to.
This will cause the associated item in the navigation bar to be highlighted.
One of: * Official SE-EDU projects only |
not set |
|
Set this attribute to remove the site navigation bar. |
not set |
4.6. Site Template
The files in docs/stylesheets
are the CSS stylesheets of the site.
You can modify them to change some properties of the site’s design.
The files in docs/templates
controls the rendering of .adoc
files into HTML5.
These template files are written in a mixture of Ruby and Slim.
Modifying the template files in |
5. Testing
5.1. Running Tests
There are three ways to run tests.
The most reliable way to run tests is the 3rd one. The first two methods might fail some GUI tests due to platform/resolution-specific idiosyncrasies. |
Method 1: Using IntelliJ JUnit test runner
-
To run all tests, right-click on the
src/test/java
folder and chooseRun 'All Tests'
-
To run a subset of tests, you can right-click on a test package, test class, or a test and choose
Run 'ABC'
Method 2: Using Gradle
-
Open a console and run the command
gradlew clean allTests
(Mac/Linux:./gradlew clean allTests
)
See UsingGradle.adoc for more info on how to run tests using Gradle. |
Method 3: Using Gradle (headless)
Thanks to the TestFX library we use, our GUI tests can be run in the headless mode. In the headless mode, GUI tests do not show up on the screen. That means the developer can do other things on the Computer while the tests are running.
To run tests in headless mode, open a console and run the command gradlew clean headless allTests
(Mac/Linux: ./gradlew clean headless allTests
)
5.2. Types of tests
We have two types of tests:
-
GUI Tests - These are tests involving the GUI. They include,
-
System Tests that test the entire App by simulating user actions on the GUI. These are in the
systemtests
package. -
Unit tests that test the individual components. These are in
seedu.jxmusic.ui
package.
-
-
Non-GUI Tests - These are tests not involving the GUI. They include,
-
Unit tests targeting the lowest level methods/classes.
e.g.seedu.jxmusic.commons.StringUtilTest
-
Integration tests that are checking the integration of multiple code units (those code units are assumed to be working).
e.g.seedu.jxmusic.storage.StorageManagerTest
-
Hybrids of unit and integration tests. These test are checking multiple code units as well as how the are connected together.
e.g.seedu.jxmusic.logic.LogicManagerTest
-
5.3. Troubleshooting Testing
Problem: HelpWindowTest
fails with a NullPointerException
.
-
Reason: One of its dependencies,
HelpWindow.html
insrc/main/resources/docs
is missing. -
Solution: Execute Gradle task
processResources
.
6. Dev Ops
6.1. Build Automation
See UsingGradle.adoc to learn how to use Gradle for build automation.
6.2. Continuous Integration
We use Travis CI and AppVeyor to perform Continuous Integration on our projects. See UsingTravis.adoc and UsingAppVeyor.adoc for more details.
6.3. Coverage Reporting
We use Coveralls to track the code coverage of our projects. See UsingCoveralls.adoc for more details.
6.4. Documentation Previews
When a pull request has changes to asciidoc files, you can use Netlify to see a preview of how the HTML version of those asciidoc files will look like when the pull request is merged. See UsingNetlify.adoc for more details.
6.5. Making a Release
Here are the steps to create a new release.
-
Update the version number in
MainApp.java
. -
Generate a JAR file using Gradle.
-
Tag the repo with the version number. e.g.
v0.1
-
Create a new release using GitHub and upload the JAR file you created.
6.6. Managing Dependencies
A project often depends on third-party libraries. For example, Address Book depends on the Jackson library for XML parsing. Managing these dependencies can be automated using Gradle. For example, Gradle can download the dependencies automatically, which is better than these alternatives.
a. Include those libraries in the repo (this bloats the repo size)
b. Require developers to download those libraries manually (this creates extra work for developers)
Appendix A: Product Scope
Target user profile:
-
has a need to listen to music on computers
-
prefer desktop apps over other types
-
can type fast
-
prefers typing over mouse input
-
is reasonably comfortable using CLI apps
Value proposition: listen to music and manage playlists faster than a typical mouse/GUI driven app
Appendix B: User Stories
Priorities: High (must have) - * * *
, Medium (nice to have) - * *
, Low (unlikely to have) - *
Priority | As a … | I want to … | So that I can…. |
---|---|---|---|
|
user |
Add a track |
Save them to my library |
|
user |
Delete a track |
Refine my library |
|
user with multiple playlists |
Manage playlists |
Maintain different playlists |
|
user with multiple tracks |
Manage tracks in the playlist |
Customise playlists |
|
user |
play tracks |
Listen to them |
|
user |
Pause a track |
Stop when I need to |
|
user |
Continue from pause |
Continue from set position with current track |
|
user |
Seek a track |
Skip parts of a track |
|
user |
Skip a track |
Iterate through my playlist and play a track I want to hear |
|
user |
Control volume |
Listen comfortably |
|
user |
Search for a track |
Find and a particular track with ease |
|
user |
Repeat a playlist |
Continue listening when playlist has finished |
|
user |
Shuffle a playlist |
Listen to playlists in different order |
|
user |
See usage instructions |
Refer to instructions when I need help |
Appendix C: Use Cases
(For all use cases below, the System is the JxMusic
and the Actor is the user
, unless specified otherwise)
Use case: List all playlists
MSS
-
User requests to list playlists.
-
System displays names of all playlists in library.
Use case ends.
Use case: Search for a playlist
MSS
-
User enters the command
search
. -
System displays "Enter playlist name:".
-
User enters a sequence of characters.
-
System displays all playlists that include the sequence of characters, in lexicographical order.
Use case ends.
Extensions
-
4a. There is no list that matches the name.
-
4a1. System displays "not found" message.
Use case ends.
-
Use case: Create playlist
MSS
-
User requests to create a new playlist.
-
System creates a new playlist and saves it to library.
Use case ends.
Extensions
-
2a. The name of the playlist has existed.
-
2a1. System displays an error message.
Use case ends.
-
Use case: Delete a playlist
MSS
-
User requests to delete a playlist.
-
System deletes the playlist.
Use case ends.
Extensions
-
2a. Playlist is not found.
-
2a1. System displays an error message.
Use case ends.
-
-
2b. Playlist is currently being played.
-
2a1. System stops the playing and deletes the playlist.
Use case ends.
-
Use case: Add a track into playlist
MSS
-
User requests to add a track into a playlist.
-
System searches for the track.
-
System searches for the playlist.
-
System adds the track to the playlist.
-
System displays successful message.
Use case ends.
Extensions
-
2a. The track does not exist.
-
2a1. System displays an error message.
Use case ends.
-
-
3a. The playlist does not exist.
-
3a1. System displays an error message.
Use case ends.
-
Use case: Delete a track from playlist
MSS
-
User requests to delete a track from a playlist.
-
System deletes the track.
Use case ends.
Extensions
-
2a. The track does not exist.
-
2a1. System displays an error message.
Use case ends.
-
Use case: Play a track
MSS
-
User requests to play.
-
System plays the current track.
Use case ends.
Extensions
-
2a. No track is in current playlist.
-
2a1. System goes back to the status before step 1.
Use case ends.
-
Use case: Play a playlist
MSS
-
User requests to play a playlist.
-
System searches for the playlist.
-
System plays the tracks of the playlist.
Use case ends.
Extensions
-
2a. The playlist is not found.
-
2a1. System displays an error message.
Use case ends.
-
-
3a. The playlist is empty.
Use case ends.
Use case: Pause the playing of track and resume the play
MSS
-
User requests to pause the playing of track.
-
System pauses the playing.
-
User requests to resume the playing of track.
-
System plays the track from the point it was paused.
Use case ends.
Use case: Stop the playing of track
MSS
-
User requests to stop the playing of track
-
System exits the playing of track.
Use case ends.
Use case: Seek time point in a track
MSS
-
User requests to seek the play timeline to a certain point.
-
System jumps to the point and plays from the point.
Use case ends.
Extensions
-
2a. The time point is outside the range of timeline.
-
2a1. System displays an error message and pauses current playing.
Use case ends.
-
Use case: Step forward/backward in a track
MSS
-
User requests to step forward/backward a range of time in the playing of current track.
-
System jumps to the point and plays from the point.
Use case ends.
Extensions
-
2a. The time point is outside the range of timeline.
-
2a1. System displays an error message and pauses current playing.
Use case ends.
-
Use case: Replay a track
MSS
-
User requests to replay the track.
-
System plays the track from the beginning.
Use case ends.
Use case: Navigate playlist
MSS
-
User requests to skip the next/previous track.
-
System goes to the next/previous track and plays the track.
Use case ends.
Extensions
-
2a. The next/previous track does not exist.
-
2a1. If "repeat playlist" function is on, system goes to the beginning/ending track of the playlist and plays the track.
Use case ends.
2a2. If "repeat playlist" is off, system pauses current playing.
Use case ends.
-
Use case: Switch playing modes
MSS
-
User requests to repeat track.
-
System changes to "repeat track" mode and current track will be played repeatedly.
-
User requests to repeat playlist.
-
System changes to "repeat playlist" mode and the current playlist will start over after playing all tracks.
-
User enters the command
repeat off
. -
System changes to "repeat off" mode and the play will stop after playing all the tracks.
Use case ends.
Use case: Shuffle playlist
MSS
-
User requests to shuffle the playlist.
-
System randomly reorders the sequence of tracks inside the playlist.
Use case ends.
Appendix D: Non Functional Requirements
-
Should work on any mainstream OS as long as it has Java
9
or higher installed. -
Should only support .mp3 file.
-
Should be able to hold up to 1000 playlists and 1000 tracks in total without a noticeable sluggishness in performance for typical usage.
-
A user with above average typing speed for regular English text (i.e. not code, not system admin commands) should be able to accomplish most of the tasks faster using commands than using the mouse.
Appendix F: Instructions for Manual Testing
Given below are instructions to test the app manually.
These instructions only provide a starting point for testers to work on; testers are expected to do more exploratory testing. |
Positive test cases (for normal usage scenario) are in green and negative test cases (for usage scenarios trying to break the app) are in red.
F.1. Launch
-
Initial launch
-
Download the jar file and copy into an empty folder
-
Open the terminal/command prompt/powershell
-
Enter command
java -jar jxmusic.jar
Expected: a “library” folder to be generated next to the jar file and shows GUI with sample playlists (as shown below)-
Fallback 1: enter command
unzip jxmusic.jar "library/*"
then double click the jar file -
Fallback 2: download and unzip library.zip in the folder containing the jar file, then double click the jar file
-
-
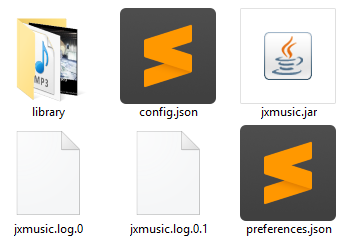
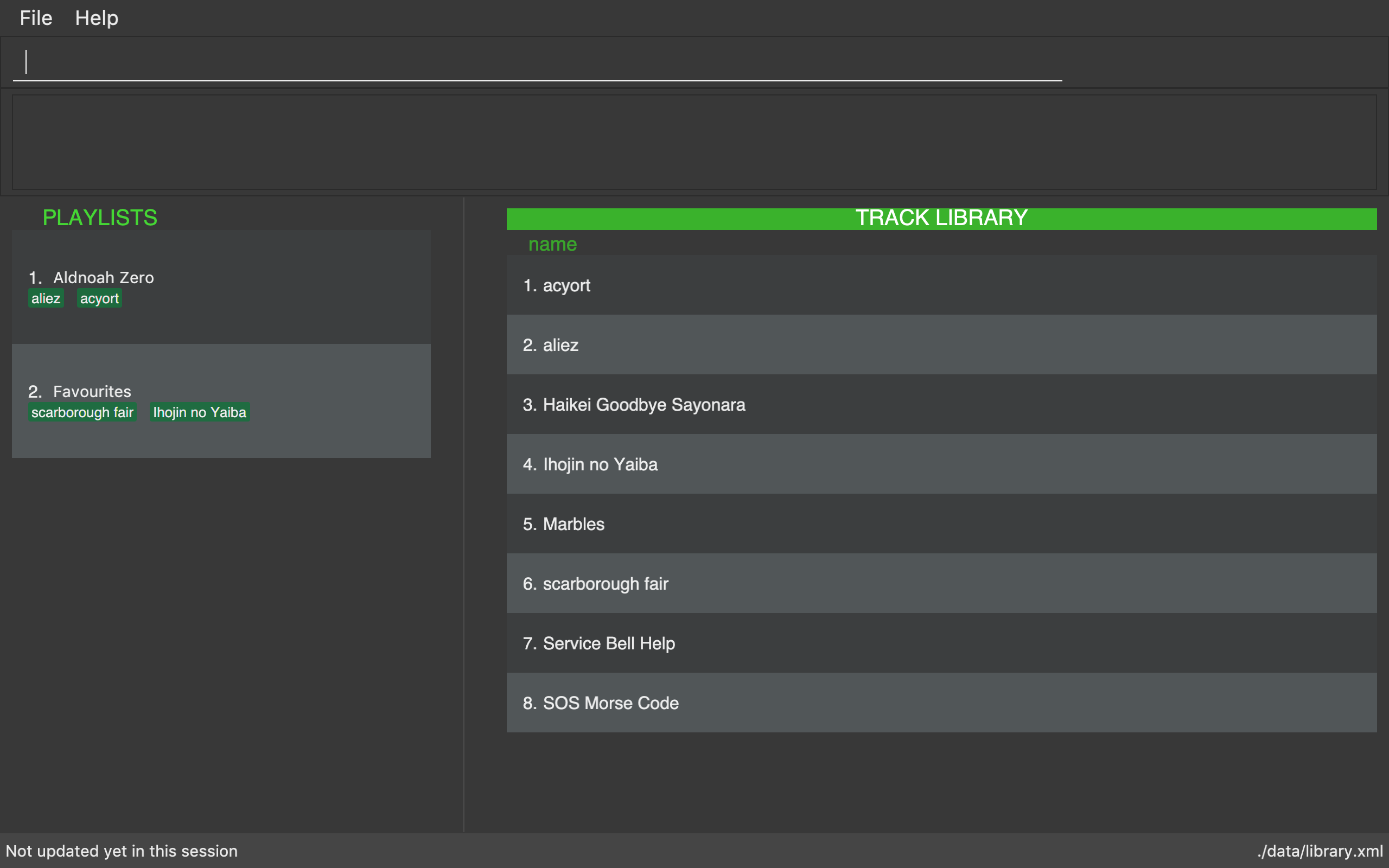
Once library folder exists and it contains the sample mp3 files, subsequent launches can be done by double clicking the jar file. |
-
Add mp3 files into the library
-
Test case: Copy and paste any of the mp3 file in the library folder, then run the jar file.
Expected: The new track file name appears in the track panel.
-
-
Add invalid mp3 file into the library
-
Test case: Save a text file as "invalid.mp3" in the library folder, then run the jar file.
Expected: "invalid" does not show up in track panel.
-
F.2. Playing tracks
You may refer Section 3.1.1.2, “PlayableStatus” for easier understanding of the possible state transitions.
-
Play the tracks in a specific playlist
-
Test case:
play p/sound effects
Expected: Tracks in "sound effects" playlist start playing sequentially.The tracks of "sound effects" playlist ("Marbles", "SOS Morse Code", "Service Bell Help") are short (few seconds in duration) sample tracks provided for testing this case.
-
-
Play the tracks in the first playlist of the library
-
Test case:
play p/
Expected: Tracks in the first playlist sorted by its name in the library starts playing.The command works even when no playlist is displayed in the panel. The first playlist in the library is the first one in the playlist panel when playlist list
is entered.
-
-
Play a specific track
-
Test case:
play t/Marbles
Expected: "Marbles" start playing.
-
-
Play a default track
-
Test case:
play t/
Expected: The first track sorted by its name in the library starts playing.Similarly to play p/
, the command works even when no track is displayed in the panel. The first track in the library is the first one in the track panel whentrack list
is entered.
-
-
Play another playlist/track while a playlist/track is playing
-
Test case:
play t/Marbles
,play t/acyort
Expected: "Marbles" stops playing and "acyort" starts playing.
-
-
Play an empty playlist
-
Prerequisites: Create an empty playlist with the
playlist new
command -
Test case:
playlist new p/empty
,play p/
Expected: Displays "playlist has no track" error message.
-
F.3. Pausing
-
Pause a playing playlist/track
-
Prerequisites: Play a playlist/track with the
play p/[playlist]
orplay t/[track]
command -
Test case:
play p/
,pause
Expected: The playing track is paused.
-
-
Pause a stopped playlist/track
-
Prerequisites: Stop a playlist/track with the
stop
command -
Test case:
play p/
,stop
,pause
Expected: Displays "no playing track to pause" error message.
-
-
Pause without playing
-
Prerequisites: No play command has been entered. Restart app for testing.
-
Test case:
pause
Expected: Displays "no playing track to pause" error message.
-
F.4. Unpausing
-
Unpause a paused playlist/track
-
Prerequisites: Pause a playing playlist/track with the
pause
command -
Test case:
play p/
,pause
,play
Expected: The paused playlist/track resumes playing from where it was paused.
-
-
Unpause a stopped playlist/track
-
Prerequisites: Stop a playing/paused track with the
stop
command -
Test case:
play p/
,stop
,play
Expected: The stopped playlist restarts playing from the first track. -
Test case:
play t/
,stop
,play
Expected: The stopped track restarts playing from the beginning.
-
-
Unpause while playing
-
Test case:
play p/
,play
Expected: Stays playing.
-
-
Unpause without playing
-
Prerequisites: No play command has been entered. Restart app for testing.
-
Test case:
play
Expected: Displays "no track paused/stopped" error message.
-
F.5. Stopping
-
Stop a playing/paused playlist/track
-
Test case:
play p/
,stop
Expected: The playing playlist/track stops playing. -
Test case:
play p/
,pause
,stop
Expected: The paused playlist/track stops playing.
-
-
Stop while stopped
-
Test case:
play p/
,stop
,stop
Expected: Stays stopped.
-
-
Stop without playing
-
Prerequisites: No play command has been entered. Restart app for testing.
-
Test case:
stop
Expected: Displays "no track to stop" error message.
-
F.6. Viewing duration of track
Due to a limitation of the media player library, duration of a track can only be made known once it starts playing. |
-
View duration of a playing/paused/stopped track
-
Test case:
play p/
,duration
Expected: Displays duration of the currently playing track. -
Test case:
play p/
,pause
,duration
Expected: Displays duration of the currently playing track. -
Test case:
play p/
,stop
,duration
Expected: Displays duration of the currently playing track.
-
-
View duration without playing
-
Prerequisites: No play command has been entered. Restart app for testing.
-
Test case:
duration
Expected: Displays "no track playing/paused/stopped" error message.
-
F.7. Seeking a track
Use the duration command to view the total length of the playing track for testing the following test cases.
|
Examples of valid TIME:10 (10 sec)1 59 (1 min 59 sec)100 (100 sec = 1 min 40 sec)1 100 (1 min + 100 sec = 2 min 40 sec)1 99 99 (1 hr + 99 min + 99 sec = 2 hr 40 min 39 sec)We did not include hour long mp3 file. If you want to test that, you need to add the file into the library folder. |
-
Seek within the duration of a track ("aliez" is 4m 26s long)
-
Test case:
play t/aliez
,seek d/100
Expected: Seek to 100th second mark and continues playing. -
Test case:
play t/aliez
,seek d/4 26
Expected: Seek to last second mark and plays for 1 second. -
Test case:
play t/aliez
,pause
,seek d/100
Expected: Seek to 100th second mark and stays paused -
Test case:
play t/aliez
,stop
,seek d/100
Expected: Displays "no playing track to seek" error message.
-
-
Seek over the duration of a track
-
Test case:
play t/aliez
,seek d/4 27
Expected: Displays "time is beyond track’s duration" error message.
-
-
Seek with invalid time input
-
Test case:
play t/aliez
,seek d/-1
Expected: Displays "wrong time format" error message. -
Test case:
play t/aliez
,seek d/-1 0
Expected: Displays "wrong time format" error message. -
Test case:
play t/aliez
,seek d/0 0 0 0
Expected: Displays "wrong time format" error message.
-
-
Seek without playing
-
Prerequisites: No play command has been entered. Restart app for testing.
-
Test case:
seek d/100
Expected: Displays "no playing track to seek" error message.
-
F.8. Creating a new playlist
-
Create an empty playlist
-
Test case:
playlist new p/new playlist
Expected: An empty playlist named “new playlist” is created
-
-
Create a playlist with tracks
-
Test case:
playlist new p/new playlist 2 t/aliez
Expected: A playlist named "new playlist 2" is created, containing "aliez" track. -
Test case:
playlist new p/sound effects t/Marbles t/SOS Morse Code t/Service Bell Help
Expected: A playlist named “sound effects” is created, containing tracks “Marbles”, “SOS Morse Code” and “Service Bell Help”
-
F.9. Searching playlists and tracks
The "search" commands (ie playlist search and track search ) works the same way except one searches for playlists, the other for tracks.
|
-
Search with substring
-
Test case:
playlist search al
Expected: Only playlists with names containing "al" are shown in the playlist panel -
Test case:
track search al
Expected: Only tracks with names containing "al" are shown in the track panel
-
-
Search with multiple substrings
-
Test case:
playlist search al i
Expected: Only playlists with names containing "al" or "i" are shown in the playlist panel -
Test case:
track search al i
Expected: Only tracks with names containing "al" or "i" are shown in the track panel
-
F.10. Listing playlists and tracks
Similarly to "search" commands, "list" commands (ie playlist list and track list ) works the same way except one for playlists, the other for tracks.
|
-
List all playlists
-
Test case:
playlist list
Expected: All playlists displayed with success message
-
-
List all tracks
-
Test case:
track list
Expected: All tracks displayed with success message
-
F.11. Adding tracks to playlist
-
Add tracks by track name
-
Test case:
track add p/Favourites t/aliez
Expected: "Favourites" playlist to be added with "aliez" track. -
Test case:
track add p/Favourites t/aliez t/acyort
Expected: "Favourites" playlist to be added with "aliez" and "acyort" tracks. -
Test case:
track add p/non existing t/aliez
Expected: Displays "playlist does not exist" error message. -
Test case:
track add p/Favourites t/non existing
Expected: Displays "track file does not exist" error message.
-
-
Add tracks by track index
-
Prerequisites: List all tracks using the
track list
command. Multiple tracks in the list. -
Test case:
track add p/Favourites i/1
Expected: "Favourites" playlist to be added with first track in panel. -
Test case:
track add p/Favourites i/1 2
Expected: "Favourites" playlist to be added with first and second tracks in panel. -
Test case:
track add p/non existing i/1
Expected: Displays "playlist does not exist" error message. -
Test case:
track add p/Favourites i/999
Expected: Displays "indexes does not exist" error message.
-
F.12. Deleting tracks in a playlist
-
Delete tracks by track index in playlist
-
Test case:
track del p/Favourites i/1
Expected: First track of "Favourites" playlist is removed from playlist. -
Test case:
track del p/non existing i/1
Expected: Displays "playlist does not exist" error message. -
Test case:
track del p/Favourites i/999
Expected: Displays "playlist does not have index" error message.
-
F.13. Deleting a playlist
-
Delete a playlist by index
-
Prerequisites: List all playlists using the
playlist list
command. Multiple playlists in the list. -
Test case:
playlist del 1
Expected: First playlist shown in panel is removed. -
Test case:
playlist del 999
Expected: Displays "index provided is invalid" error message.
-